- Retrieving Some Categories as Objects
- Retrieving a given Category with Its ID
- Categories ID’s
- Get ALL the Data!
- I Lied to You
- Retrieving a Category Without Its ID
- Retrieving Several Categories
- Get All the Categories You Want
- Get Categories Associated to a Post
- Retrieving Some Specific Data
- Retrieving the ID of a Category
- Retrieving Some Strings
- Short Lists
- Conclusion
- Frequently Asked Questions about Mastering WordPress Categories API
In a previous SitePoint article, I provided an introduction to the WordPress Categories API thanks to the function wp_list_categories()
. As we saw, this function is full of different options, and that’s why it’s a good idea to use it if we want to display a list of our categories.
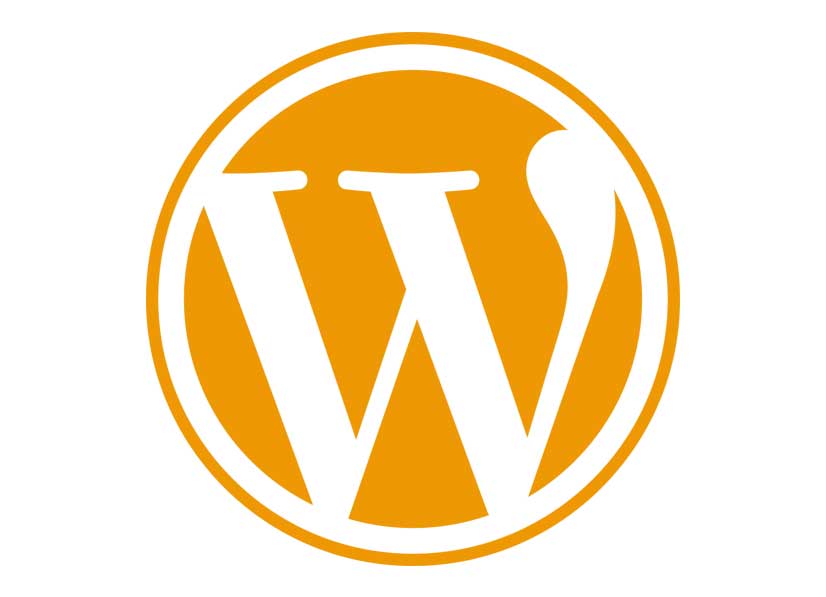
However, it can’t cover all the possible cases, and that’s the reason why we find other functions in the Categories API. These functions allow us to retrieve raw data: in place of a HTML list of our categories, we can retrieve arrays containing these same categories.
The consequence is that it’s simpler (and proper!) to display this data in a special way, whatever you can imagine as a DOM tree. We will begin here with functions that return objects containing all data relative to our categories. Then, we’ll be interested in functions that only return specific information.
Retrieving Some Categories as Objects
Like links in the Links Manager, we can retrieve our categories as objects that contain all of their properties. We’ll begin by describing these objects thanks to a function which only returns one category. Then, we’ll use our newly acquired knowledge to play with all the categories we want!
Retrieving a given Category with Its ID
The first function we’ll cover here is get_category()
. Basically, it allows you to get an object representing the category you pass the ID in as a parameter.
$cat = get_category(14);
Then, $cat
contains the wanted object. We’ll now describe some of its properties that are of interest to us.
Categories ID’s
First, the ID of the category we wanted. You can find this value in the cat_ID
property.
$id = $cat->cat_ID;
Note that this value is not very useful here, as we already knew the ID (we passed it as the parameter of the function!). But we’ll see below that there are other ways to retrieve a category: in that case, knowing how to get the ID in a category object can be useful.
If your category is a child of another one, you can also retrieve the ID of its parent thanks to the property category_parent
. Note that if your category has no parent, this value is set to 0
.
Get ALL the Data!
When we display information about a category, one essential piece of information is its name. You can retrieve it thanks to the property cat_name
. In the same way, you can retrieve the category’s description thanks to category_description
.
<h1><?php echo $cat->cat_name; ?></h1>
<p><?php echo $cat->category_description; ?></p>
The ID is not the unique identifier of a category: the slug is another one, in the shape of a string (generally the name of the category itself, in lowercase, without spaces or special characters). You can retrieve the slug with the property category_nicename
, or slug
(both are valid).
Finally, you can also see how many posts are contained into this category thanks to the property category_count
. Note that this counter doesn’t take into account the number of posts we can found in children categories.
I Lied to You
The title of this part is a lie. In fact, objects are not the only available representation of our categories. You can also choose to retrieve them in arrays, thanks to a second argument given to get_category()
.
// Get an object (default behavior)
$cat = get_category(15, OBJECT);
// Get an associative array
$cat = get_category(15, ARRAY_A);
// Get an numbered array
$cat = get_category(15, ARRAY_N);
The keys in the associative array are the properties of the object. However, things are more complicated in the numbered array: the order is the same, but you can’t have clear indexes.
Retrieving a Category Without Its ID
As we said above, ID is not the only identifier of a category, and the slug of a category can also be used to retrieve its information. This can be achieved with the function get_category_by_slug()
. As you can guess, it accepts the slug as a parameter.
$cat = get_category_by_slug('my-goldfish');
We won’t describe the returned object, as it’s the same as we had with get_category()
. However, you don’t have a choice here: object is the only available type and you won’t be able to retrieve an array with this function. Finally, note that get_category_by_slug()
returns false
if there is no category with the indicated slug.
Retrieving Several Categories
We’ll see two functions to get different lists of categories. The first function allows you to retrieve any list of categories, while the second will help you retrieve the categories associated to a given post.
Get All the Categories You Want
If you read the previous part you won’t be disoriented by the returned values of get_categories()
, as it returns an array listing all the wanted categories, each represented by an object which is exactly the one we described above.
Without any argument, this function returns all the categories you created. If you write the line below:
$cats = get_categories();
then $cats
is an array containing all your categories, in alphabetical order. As an example, if I want to retrieve the name of the second category, all I have to write is $cats[1]->cat_name
(see the previous part about the category object for more info).
If you did a print_r()
on the $cats
variable, maybe you noticed something weird: in some cases, this array has some “holes”. For example, we can directly step from index 0
to 4
, without seeing 1
, 2
and 3
. What happened? What is this weird way of counting?
Well, in fact, this is not weird: if you effectively noticed this “bug”, maybe you also noticed that the missing categories are exactly the ones which are empty: by default, WordPress hides the empty categories. Hurray, it’s not a bug!
We can modify this behavior, along with some other things we can customize thanks to the argument we can send to get_categories()
. As usual, this unique argument is an array containing all the options we want.
I recommend you to read our previous article about the Categories API, where we described wp_list_categories()
. In fact, (almost) all the available options for get_categories()
are options we saw for wp_list_categories()
, so we won’t describe what they do twice.
As for wp_list_categories()
you can choose the order you want with orderby
and order
, limit the number of returned categories with number
or again choose to get the empty categories with hide_empty
. You also have access to the options include
and exclude
to get the right categories.
Maybe the hierarchical
option deserves an explanation: set by default to 1
, you can cancel its effects by setting it to 0
. Set to 1
, this option can return some empty categories, even if hide_empty
is set to 1
. However, you’ll only see empty categories with children categories which are not empty (that way you can build the entire tree).
The pad_counts
option is still here, so is the child_of
one. But a new option is here: parent
which is like child_of
, with the difference that parent
only returns the direct children of the selected category: if these children themselves have children categories, they won’t be returned.
Get Categories Associated to a Post
You can associate more than one category to a post. When you use get_the_category()
, you can retrieve an array listing of all the categories you set for a given post.
By default, this function returns all the categories associated with the current post (you have to be in the loop to enable this behavior). However, you can retrieve the categories associated to any post by passing its ID as a parameter.
// Get the categories of the post with ID 139
$cats = get_the_category(139);
Retrieving Some Specific Data
Sometimes, we just need specific information, like the name of a category, or its ID. In these cases, retrieving an entire object to get only one lot of information is useless. That’s why there are some other functions for these specific needs.
Retrieving the ID of a Category
It’s always easier to remember the name of a category instead of its ID (which is never the same following the installation). The good news is that you can retrieve the ID of a category if you know its name. To do that we can use the get_cat_ID()
function: you pass it the name of a category and it returns its ID. If no category was found, the returned value is 0
.
// Yes, I love this category
$cat_id = get_cat_ID('My goldfish');
If you already have the ID of a category but don’t know the IDs of its parents you can also use get_ancestors()
. It returns an array containing all the ancestors’ IDs, from the nearest to the farthest.
An example will be clearer. Assume that we have “My goldfish” (with ID 15) as a child category of “My life” (with ID 14). Now we add a child to “My goldfish“: “The feed of my goldfish” (with ID 16). Then the following line:
$cats_id = get_ancestors(16, 'category');
returns this:
Array
(
[0] => 15
[1] => 14
)
Note that we have to indicate to get_ancestors()
that we’re talking about categories. In fact, this function is more general and can include more fields than categories (but it’s not what we’re interested in here).
Retrieving Some Strings
We can retrieve the ID of a category from its name, but we can also do the opposite: that’s what get_cat_name()
does.
// Returns the name of the category with ID 15
$cat_name = get_cat_name(15);
The same thing can be done with the description of a category, thanks to category_description()
.
// Returns the description of the category with ID 15
$cat_desc = category_description(15);
Did you notice that, since the beginning of this article, we haven’t talked about the URL of a category? It’s an interesting topic, as its shape can change following the installation (URL Rewriting? No URL Rewriting?). To be honest, I don’t understand why this information is not contained into the object we described above. However, we can retrieve the URL of a category thanks to get_category_link()
.
// I want to display a link to my favorite category
$cat_id = get_cat_ID('My goldfish');
$cat_link = get_category_link($cat_id);
Then all I have to do is using $cat_link
which contains the right URL.
<a href="<?php echo $cat_link; ?>">My goldfish</a>
Short Lists
If you’ve been developing WordPress themes, chances are that you already know the_category()
. This function allows you to echo a list of the categories used by the current post (or any other). This list is a string, with all the links one after the other, separated by the string you want (indicated in the first parameter).
// Get a comma-separated list of the used categories
the_category(', ');
The third parameter of this function is the post ID we want: if you’re not in the loop, you must use this parameter. The second parameter is quite particular.
It allows you to control the way relationships between parents and children are displayed and is useful only in the case where the concerned post is in a category that has a parent category. By default this parameter is set to an empty string and only the child category is shown. If you change this to single
, then the name of the parent category will also be displayed, in the same link of its child. Finally, you can also choose multiple
: with this value, the parent and the child category are shown, in two separate links.
If you prefer storing the result into a variable before echoing it, then you can use get_the_category_list()
instead (it can be used in the same way than the_category()
).
Conclusion
The Categories API is a very comprehensive, it contains many very useful functions. Whatever you need when you want to play with categories, chances are great that a function already exists.
In fact, I chose to describe some of the most commonly used functions, but this API contains a large number of others. For example, there’s a function to check if a post is in a category. There’s also a function that displays a list composed by checkboxes for every category (useful for administration panels).
Describing all of them will be more indigestible than anything else. That’s why, if you can’t find the function in this article, don’t hesitate to ask for help below.
Frequently Asked Questions about Mastering WordPress Categories API
How can I retrieve a list of categories in WordPress REST API?
To retrieve a list of categories in WordPress REST API, you need to make a GET request to the ‘/wp/v2/categories’ endpoint. This will return a JSON object containing all the categories on your WordPress site. Each category in the list will have properties like id, count, description, link, name, slug, taxonomy, and parent. You can use these properties to display the categories in your application or filter posts by category.
How can I add a new category using the WordPress REST API?
To add a new category using the WordPress REST API, you need to make a POST request to the ‘/wp/v2/categories’ endpoint. In the body of the request, you should include a JSON object with the properties of the new category. The ‘name’ property is required, but you can also include optional properties like ‘description’ and ‘parent’. After the request is successful, the API will return a JSON object with the details of the new category.
How can I update a category using the WordPress REST API?
To update a category using the WordPress REST API, you need to make a POST request to the ‘/wp/v2/categories/
How can I delete a category using the WordPress REST API?
To delete a category using the WordPress REST API, you need to make a DELETE request to the ‘/wp/v2/categories/
How can I retrieve a specific category using the WordPress REST API?
To retrieve a specific category using the WordPress REST API, you need to make a GET request to the ‘/wp/v2/categories/
How can I retrieve posts from a specific category using the WordPress REST API?
To retrieve posts from a specific category using the WordPress REST API, you need to make a GET request to the ‘/wp/v2/posts’ endpoint with a ‘categories’ parameter set to the ID of the category. This will return a JSON object with the posts in the category.
How can I retrieve the parent of a category using the WordPress REST API?
To retrieve the parent of a category using the WordPress REST API, you need to make a GET request to the ‘/wp/v2/categories/
How can I retrieve the children of a category using the WordPress REST API?
To retrieve the children of a category using the WordPress REST API, you need to make a GET request to the ‘/wp/v2/categories’ endpoint with a ‘parent’ parameter set to the ID of the category. This will return a JSON object with the children of the category.
How can I retrieve the count of posts in a category using the WordPress REST API?
To retrieve the count of posts in a category using the WordPress REST API, you need to make a GET request to the ‘/wp/v2/categories/
How can I retrieve the slug of a category using the WordPress REST API?
To retrieve the slug of a category using the WordPress REST API, you need to make a GET request to the ‘/wp/v2/categories/
Currently a math student, Jérémy is a passionate guy who is interested in many fields, particularly in the high tech world for which he covers the news everyday on some blogs, and web development which takes much of his free time. He loves learning new things and sharing his knowledge with others.