If your using the jQuery validation plugin you might sometimes see a stutter validation when your typing in an input field. This is most commonly seen when your using custom validation rules which are firing off ajax requests to validate the users input, such as checking if a users email is unique in the database. Stutter = no good. To remove the consant validation checking add the following parameters to the form validate function:
onkeyup: false,
onclick: false,
onfocusout: false,
So your validation function might look something like this:
$("#form").validate(
{
onkeyup: false,
onclick: false,
onfocusout: false,
//validation rules
rules: {
...
},
//validation messages
messages: {
...
},
//submit handler
submitHandler: function(form)
{
...
}
})
Other $.validate() options
messages: {},
groups: {},
rules: {},
errorClass: "error",
validClass: "valid",
errorElement: "label",
focusInvalid: true,
errorContainer: $( [] ),
errorLabelContainer: $( [] ),
onsubmit: true,
ignore: ":hidden",
ignoreTitle: false,
onfocusin: function(element, event) { ... },
onfocusout: function(element, event) { ... },
onkeyup: function(element, event) { ... },
onclick: function(element, event) { ... },
highlight: function(element, errorClass, validClass) { ... },
unhighlight: function(element, errorClass, validClass) { ... }
Frequently Asked Questions (FAQs) about jQuery Validation and Form Submission
How Can I Customize Error Messages in jQuery Validation?
jQuery Validation plugin allows you to customize error messages according to your needs. You can do this by using the messages
option in the .validate()
method. Here’s an example:$("#myForm").validate({
rules: {
name: {
required: true,
minlength: 2
}
},
messages: {
name: {
required: "Please enter your name",
minlength: "Your name must consist of at least 2 characters"
}
}
});
In this example, if the ‘name’ field is left empty, the custom error message “Please enter your name” will be displayed. If the ‘name’ field has less than 2 characters, “Your name must consist of at least 2 characters” will be shown.
How Can I Use jQuery Validation for Multiple Forms on the Same Page?
You can use jQuery Validation for multiple forms on the same page by calling the .validate()
method on each form separately. Here’s an example:$("#form1").validate({
// rules and messages for form1
});
$("#form2").validate({
// rules and messages for form2
});
In this example, #form1
and #form2
are the IDs of the two forms. You can define separate validation rules and messages for each form.
How Can I Use jQuery Validation with AJAX?
You can use jQuery Validation with AJAX by using the submitHandler
option in the .validate()
method. The submitHandler
function is called when the form is valid, and it is where you can submit your form via AJAX. Here’s an example:$("#myForm").validate({
rules: {
// your rules
},
messages: {
// your messages
},
submitHandler: function(form) {
$.ajax({
type: "POST",
url: "/your-url",
data: $(form).serialize(),
success: function(response) {
// handle the response
}
});
}
});
In this example, when the form is valid, it is submitted via AJAX to the URL “/your-url”. The $(form).serialize()
method is used to create a text string in standard URL-encoded notation.
How Can I Validate a Specific Field with jQuery Validation?
You can validate a specific field with jQuery Validation by using the rules
option in the .validate()
method. Here’s an example:$("#myForm").validate({
rules: {
email: {
required: true,
email: true
}
}
});
In this example, the ’email’ field is required and it must be a valid email address.
How Can I Disable jQuery Validation for Certain Fields?
You can disable jQuery Validation for certain fields by using the ignore
option in the .validate()
method. Here’s an example:$("#myForm").validate({
ignore: ".ignore"
});
In this example, all fields with the class ‘ignore’ will be ignored by the validation.
How Can I Use jQuery Validation with Radio Buttons and Checkboxes?
You can use jQuery Validation with radio buttons and checkboxes by using the required
rule. Here’s an example:$("#myForm").validate({
rules: {
"gender": {
required: true
},
"interests": {
required: true
}
}
});
In this example, the ‘gender’ radio buttons and the ‘interests’ checkboxes are required.
How Can I Validate a Field Based on the Value of Another Field with jQuery Validation?
You can validate a field based on the value of another field with jQuery Validation by using the addMethod()
function to create a custom validation method. Here’s an example:$.validator.addMethod("greaterThan", function(value, element, param) {
return this.optional(element) || parseInt(value) > parseInt($(param).val());
}, "Must be greater than {0}");
$("#myForm").validate({
rules: {
field1: {
required: true,
number: true
},
field2: {
required: true,
number: true,
greaterThan: "#field1"
}
}
});
In this example, ‘field2’ must be greater than ‘field1’.
How Can I Validate a Field Only If It Is Filled with jQuery Validation?
You can validate a field only if it is filled with jQuery Validation by using the required
rule with a function. Here’s an example:$("#myForm").validate({
rules: {
email: {
required: function(element) {
return $("#name").val() != "";
},
email: true
}
}
});
In this example, the ’email’ field is required only if the ‘name’ field is filled.
How Can I Validate a Field Based on a Regular Expression with jQuery Validation?
You can validate a field based on a regular expression with jQuery Validation by using the addMethod()
function to create a custom validation method. Here’s an example:$.validator.addMethod("regex", function(value, element, regexp) {
return this.optional(element) || regexp.test(value);
}, "Please enter a valid value.");
$("#myForm").validate({
rules: {
name: {
regex: /^[a-zA-Z\s]*$/
}
}
});
In this example, the ‘name’ field must match the regular expression /^[a-zA-Z\s]*$/
, which allows only letters and spaces.
How Can I Validate a Field for a Minimum and Maximum Number with jQuery Validation?
You can validate a field for a minimum and maximum number with jQuery Validation by using the min
and max
rules. Here’s an example:$("#myForm").validate({
rules: {
age: {
required: true,
number: true,
min: 18,
max: 99
}
}
});
In this example, the ‘age’ field is required, it must be a number, and it must be between 18 and 99.
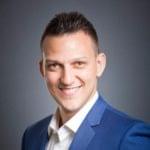
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.