Simple jQuery code snippet to remove an entire table column based on the column number. It also removes the table row heading associated with the removed column.
//remove the 1st column
$('#table').find('td,th').first().remove();
//remove the 1st column
$('table tr').find('td:eq(1),th:eq(1)').remove();
//remove the 2nd column
$('table tr').find('td:eq(1),th:eq(1)').remove();
//remove the nth column
$('table tr').find('td:eq(n),th:eq(n)').remove();
Frequently Asked Questions (FAQs) about jQuery Table Column Removal
How can I remove a specific column from a table using jQuery?
To remove a specific column from a table using jQuery, you need to use the :nth-child()
selector. This selector matches every element that is the nth child, regardless of type, of its parent. Here’s an example of how you can use it:$("table td:nth-child(2),th:nth-child(2)").remove();
In this example, the second column of the table will be removed. You can replace ‘2’ with the number of the column you want to remove.
Can I remove multiple columns from a table using jQuery?
Yes, you can remove multiple columns from a table using jQuery. You just need to specify the column numbers in the :nth-child()
selector. Here’s an example:$("table td:nth-child(2),th:nth-child(2),td:nth-child(3),th:nth-child(3)").remove();
In this example, the second and third columns of the table will be removed.
How can I hide a column instead of removing it?
If you want to hide a column instead of removing it, you can use the hide()
method in jQuery. Here’s an example:$("table td:nth-child(2),th:nth-child(2)").hide();
In this example, the second column of the table will be hidden.
Can I use jQuery to remove a column based on its content?
Yes, you can use jQuery to remove a column based on its content. You can use the :contains()
selector to find the column with the specific content and then use the remove()
method to remove it. Here’s an example:$("table td:contains('Content'),th:contains('Content')").remove();
In this example, any column containing the word ‘Content’ will be removed.
How can I remove a column from a table without using jQuery?
If you don’t want to use jQuery, you can use plain JavaScript to remove a column from a table. Here’s an example:var table = document.getElementById("myTable");
for (var i = 0; i < table.rows.length; i++) {
table.rows[i].deleteCell(1);
}
In this example, the second column of the table will be removed. You can replace ‘1’ with the index of the column you want to remove.
How can I remove the last column of a table using jQuery?
To remove the last column of a table using jQuery, you can use the :last-child
selector. Here’s an example:$("table td:last-child,th:last-child").remove();
In this example, the last column of the table will be removed.
Can I remove a column from a table using jQuery based on its header?
Yes, you can remove a column from a table using jQuery based on its header. You can use the :contains()
selector to find the header with the specific content and then use the remove()
method to remove the corresponding column. Here’s an example:var index = $("table th:contains('Header')").index() + 1;
$("table td:nth-child(" + index + "),th:nth-child(" + index + ")").remove();
In this example, the column with the header ‘Header’ will be removed.
How can I remove a column from a table using jQuery if I don’t know its number?
If you don’t know the number of the column you want to remove, you can use the :contains()
selector to find the column with the specific content and then use the remove()
method to remove it. Here’s an example:$("table td:contains('Content'),th:contains('Content')").remove();
In this example, any column containing the word ‘Content’ will be removed.
Can I remove a column from a table using jQuery and then add it back?
Yes, you can remove a column from a table using jQuery and then add it back. However, once a column is removed, it cannot be added back directly. You need to store the column in a variable before removing it and then append it back when needed.
How can I remove a column from a table using jQuery without affecting the layout?
When you remove a column from a table using jQuery, the layout of the table will be automatically adjusted. However, if you want to maintain the layout, you can hide the column instead of removing it. Here’s an example:$("table td:nth-child(2),th:nth-child(2)").hide();
In this example, the second column of the table will be hidden, and the layout of the table will be maintained.
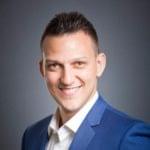
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.