This is how you might go about using the new HTML5 Custom Data Attributes feature available in HTML5 with the jQuery.data() function. It is very useful for adding data into a page and passing custom settings for DOM elements into JavaScript for some initialisation code at runtime.
HTML5 with Custom Data Attributes
Considering the following div I have added some random data attributes. It’s basically just prepend any identifier with “data-” and don’t include quotes on booleans.
Lorem ipsum dolor sit amet, consectetur adipiscing elit...
Using jQuery.Data();
Here is different ways to get the data from the DOM element using jQuery (in a document ready).$('.widget').data('name'); // "Sam Deering"
$('.widget').data().name; // "Sam Deering"
$('.widget').data(); // Object { noob=false, man=true, favFood="pizza", more...}
//identifier with mutiple words (ie data-fav-food="pizza")
$('.widget').data('favFood'); // pizza
//identifier with boolean value (ie noob=false)
$('.widget').data('noob'); // false
Try it yourself
I created a jQuery.Data() jsFiddle for you to play around with the data attributes and do some testing.Basic Example
In the following example I are using the data attibutes to set the title, size, position and behaviour of a div element. The first div has also been given drag and resize privileges.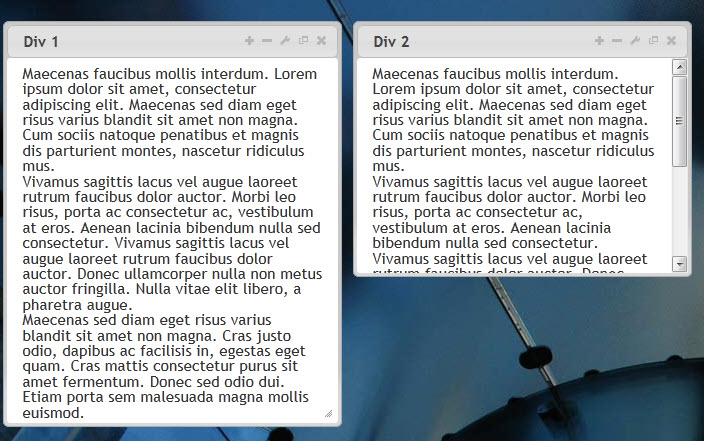
HTML
Maecenas faucibus mollis interdum. Lorem ipsum dolor sit amet, consectetur adipiscing elit. Maecenas sed diam eget risus varius blandit sit amet non magna. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus.
Vivamus sagittis lacus vel augue laoreet rutrum faucibus dolor auctor. Morbi leo risus, porta ac consectetur ac, vestibulum at eros. Aenean lacinia bibendum nulla sed consectetur. Vivamus sagittis lacus vel augue laoreet rutrum faucibus dolor auctor. Donec ullamcorper nulla non metus auctor fringilla. Nulla vitae elit libero, a pharetra augue.
Maecenas sed diam eget risus varius blandit sit amet non magna. Cras justo odio, dapibus ac facilisis in, egestas eget quam. Cras mattis consectetur purus sit amet fermentum. Donec sed odio dui. Etiam porta sem malesuada magna mollis euismod.
Maecenas faucibus mollis interdum. Lorem ipsum dolor sit amet, consectetur adipiscing elit. Maecenas sed diam eget risus varius blandit sit amet non magna. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus.
Vivamus sagittis lacus vel augue laoreet rutrum faucibus dolor auctor. Morbi leo risus, porta ac consectetur ac, vestibulum at eros. Aenean lacinia bibendum nulla sed consectetur. Vivamus sagittis lacus vel augue laoreet rutrum faucibus dolor auctor. Donec ullamcorper nulla non metus auctor fringilla. Nulla vitae elit libero, a pharetra augue.
Maecenas sed diam eget risus varius blandit sit amet non magna. Cras justo odio, dapibus ac facilisis in, egestas eget quam. Cras mattis consectetur purus sit amet fermentum. Donec sed odio dui. Etiam porta sem malesuada magna mollis euismod.
JQUERY
//loop for each
...
//defaults settings (get from data attributes)
var d_all = elem.data(),
d_title = (d_all.title) ? d_all.title : "Div Title";
d_left = (d_all.left >= 0) ? d_all.left : 50,
d_top = (d_all.top >= 0) ? d_all.top : 50,
d_w = (d_all.width >= 0) ? d_all.width : 600,
d_h = (d_all.height >= 0) ? d_all.height : 350,
d_resize = (d_all.resize) ? d_all.resize : false,
d_drag = (d_all.drag) ? d_all.drag : false;
//create the dialog using settings
elem.dialog(
{
"title": d_title,
"height": d_h,
"width": d_w,
"position": [ d_left, d_top ],
"resizable": d_resize,
"draggable": d_drag
});
Further reading into jQuery.data();
Where does this data get stored?
You can also pass objects when setting new data instead of key/value pairs. An object can be passed to jQuery.data instead of a key/value pair; this gets shallow copied over onto the existing cache.//https://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.js Line: 1741
if ( typeof name === "object" || typeof name === "function" ) {
if ( pvt ) {
cache[ id ] = jQuery.extend( cache[ id ], name );
} else {
cache[ id ].data = jQuery.extend( cache[ id ].data, name );
}
}
Where does this data get stored?
jQuery data() is stored in a separate object inside the object’s internal data cache in order to avoid key collisions between internal data and user-defined data.//https://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.js Line: 1753
if ( !pvt ) {
if ( !thisCache.data ) {
thisCache.data = {};
}
thisCache = thisCache.data;
}
What about boolean and integer types?
Play around with it: https://jsfiddle.net/KMThA/3/HTML
Lorem ipsum dolor sit amet, consectetur adipiscing elit...
jQuery
var customData = $('.widget').data();
console.dir(customData);
$.each(customData, function(i, v)
{
console.log(i + ' = ' + v + ' (' + typeof(v) + ')'); // name = value (type)
});
Output
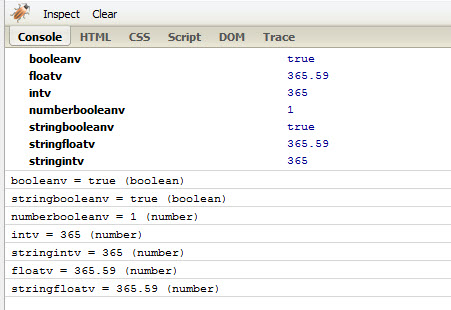
Frequently Asked Questions about jQuery Data and HTML5 Custom Data Attributes
How can I use jQuery to manipulate HTML5 custom data attributes?
jQuery provides a simple and efficient way to manipulate HTML5 custom data attributes. You can use the .data()
method to get the value of a data attribute. For instance, if you have an element with a data attribute like <div data-role="page">
, you can get the value of the ‘role’ data attribute using $('div').data('role')
. Similarly, you can set the value of a data attribute using the same .data()
method. For example, $('div').data('role', 'newRole')
will change the value of the ‘role’ data attribute to ‘newRole’.
What is the difference between .data()
and .attr()
in jQuery?
Both .data()
and .attr()
methods in jQuery can be used to get or set data-attribute values. However, there is a significant difference between the two. The .data()
method allows you to attach data of any type to DOM elements in a way that is safe from circular references and therefore from memory leaks. On the other hand, the .attr()
method gets the attribute value for only the first element in the matched set. It returns undefined for values of undefined elements and it sets the attribute for only the matched set.
How can I remove data attributes using jQuery?
You can remove data attributes using the .removeAttr()
method in jQuery. For instance, if you have an element with a data attribute like <div data-role="page">
, you can remove the ‘role’ data attribute using $('div').removeAttr('data-role')
. Please note that this will completely remove the attribute from the DOM element.
Can I use jQuery to add multiple data attributes at once?
Yes, you can use jQuery to add multiple data attributes at once. You can use the .data()
method and pass an object with key-value pairs. For example, $('div').data({role: 'page', id: 'unique'})
will add two data attributes, ‘role’ and ‘id’, to the ‘div’ element.
How can I select elements based on data attribute values in jQuery?
You can use the attribute selector in jQuery to select elements based on data attribute values. For instance, if you want to select all div elements with a ‘role’ data attribute value of ‘page’, you can use $('div[data-role="page"]')
.
Are there any performance considerations when using jQuery data methods?
Yes, there are performance considerations when using jQuery data methods. Accessing data attributes via jQuery’s .data()
method can be slower than accessing them directly via JavaScript’s native getAttribute()
method. However, the difference is usually negligible unless you’re performing these operations thousands of times.
Can I use jQuery data methods with JSON data?
Yes, you can use jQuery data methods with JSON data. You can store a JSON object as a data attribute value and then retrieve it using the .data()
method. However, you need to use JSON.stringify()
when setting the data attribute value and JSON.parse()
when retrieving it.
How can I use jQuery to get all data attributes of an element?
You can use the .data()
method without any arguments to get all data attributes of an element. For example, $('div').data()
will return an object containing all data attributes of the ‘div’ element.
Can I use jQuery data methods with arrays?
Yes, you can use jQuery data methods with arrays. You can store an array as a data attribute value and then retrieve it using the .data()
method. However, you need to use JSON.stringify()
when setting the data attribute value and JSON.parse()
when retrieving it.
Are jQuery data methods case-sensitive?
Yes, jQuery data methods are case-sensitive. For instance, $('div').data('role')
and $('div').data('Role')
will return different results. The former will return the value of the ‘role’ data attribute, while the latter will return undefined unless there is a ‘Role’ data attribute.
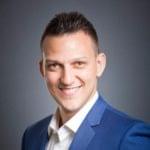
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.