I only just found out that the jQuery validation plugins has a validation rule called “remote” which can be used to make an ajax request instead of specifying a custom rule which has an ajax call it in. This will save you heaps of time with those custom validation rules. Basic jQuery Form Validation Tutorial (2mins). Example: Checking if a users email is already registered.
The new remote method way
As you can see to pass through data you can simply use the key pair syntax so the request sent below the data is “&emails=email@jquery4u.com”. The return values for your backend script is either json encoded true for a validation pass or html msg for validation fail.//VALIDATE USER EMAIL
$(':input[name="uAcc"]').rules("add",
{
"remote" :
{
url: 'validateEmail.php',
type: "post",
data:
{
emails: function()
{
return $('#register-form :input[name="email"]').val();
}
}
}
});
The old add custom method way
//VALIDATE USER EMAIL
$.validator.addMethod("validateUserEmail", function(value, element)
{
var inputElem = $('#register-form :input[name="email"]'),
data = { "emails" : inputElem.val() },
eReport = ''; //error report
$.ajax(
{
type: "POST",
url: validateEmail.php,
dataType: "json",
data: data,
success: function(data)
{
if (data !== 'true')
{
return 'This email address is already registered.';
}
else
{
return true;
}
},
error: function(xhr, textStatus, errorThrown)
{
alert('ajax loading error... ... '+url + query);
return false;
}
});
}, '');
$(':input[name="email"]').rules("add", { "validateUserEmail" : true} );
Backend PHP Script
< ?php
/* check if email is already registered */
//connect to db using mysqli
if (!empty($_POST['email']))
{
$email = $mysqli->real_escape_string($_POST['email']);
$query = "SELECT ID FROM users WHERE user_email = '{$email}' LIMIT 1;";
$results = $mysqli->query($query);
if($results->num_rows == 0)
{
echo "true"; //good to register
}
else
{
echo "false"; //already registered
}
}
else
{
echo "false"; //invalid post var
}
?>
Another Example to help
/* register script */
(function($,W,D,undefined)
{
$(D).ready(function()
{
//form validation rules
$("#register-form").validate({
rules:
{
email:
{
required: true,
email: true,
"remote":
{
url: 'validateEmail.php',
type: "post",
data:
{
email: function()
{
return $('#register-form :input[name="email"]').val();
}
}
}
},
name:
{
required: true,
minlength: 3
},
password:
{
required: true,
minlength: 8
},
password_repeat:
{
required: true,
equalTo: password,
minlength: 8
}
},
messages:
{
email:
{
required: "Please enter your email address.",
email: "Please enter a valid email address.",
remote: jQuery.validator.format("{0} is already taken.")
},
name: "Please enter your name.",
password: "Please enter a password.",
password_repeat: "Passwords must match."
},
submitHandler: function(form)
{
form.submit();
}
});
});
})(jQuery, window, document);
Stopping remote validation as you type
By default the validation plugin will send off an ajax request for a remote rule every key you press causing too many ajax requests being sent to validate fields. One way to disable this is to deactivate the onkeyup validation so that the remote rule is only validated via ajax once you have finished typing into the input.$("#register-form").validate({
onkeyup: false //turn off auto validate whilst typing
});
Frequently Asked Questions (FAQs) about jQuery AJAX Validation Remote Rule
What is the jQuery AJAX Validation Remote Rule?
The jQuery AJAX Validation Remote Rule is a feature in jQuery Validation Plugin that allows you to perform asynchronous validation on form fields using AJAX. This rule is particularly useful when you need to validate a field against data on the server, such as checking if a username or email is already registered. The remote rule sends an AJAX request to the server with the field value and waits for the server’s response to determine if the value is valid or not.
How do I implement the jQuery AJAX Validation Remote Rule?
To implement the jQuery AJAX Validation Remote Rule, you need to use the ‘remote’ method in the jQuery Validation Plugin. This method takes a URL as a parameter, which is the server-side script that will perform the validation. Here’s a basic example:$("#myForm").validate({
rules: {
username: {
remote: "check-username.php"
}
}
});
In this example, the ‘check-username.php’ script will receive the username value and return ‘true’ if the username is valid, or ‘false’ if it’s not.
Can I customize the AJAX request sent by the remote rule?
Yes, you can customize the AJAX request sent by the remote rule. Instead of passing a URL string to the ‘remote’ method, you can pass an object with AJAX settings. For example:$("#myForm").validate({
rules: {
username: {
remote: {
url: "check-username.php",
type: "post",
data: {
username: function() {
return $("#username").val();
}
}
}
}
}
});
In this example, the AJAX request is sent as a POST request, and the username value is included in the request data.
How can I display custom error messages with the remote rule?
You can display custom error messages with the remote rule by using the ‘messages’ option in the jQuery Validation Plugin. For example:$("#myForm").validate({
rules: {
username: {
remote: "check-username.php"
}
},
messages: {
username: {
remote: "This username is already taken."
}
}
});
In this example, if the username is not valid, the message “This username is already taken.” will be displayed.
Can I use the remote rule with other validation rules?
Yes, you can use the remote rule with other validation rules. The jQuery Validation Plugin allows you to specify multiple rules for a field. For example:$("#myForm").validate({
rules: {
username: {
required: true,
minlength: 5,
remote: "check-username.php"
}
}
});
In this example, the username field is required, must be at least 5 characters long, and must be valid according to the ‘check-username.php’ script.
How does the server-side script process the AJAX request from the remote rule?
The server-side script receives the AJAX request from the remote rule, processes the validation, and returns a response. The script should return ‘true’ if the value is valid, or ‘false’ if it’s not. The response should be in JSON format. Here’s a basic example in PHP:<?php
$username = $_POST['username'];
// Perform validation...
if ($isValid) {
echo 'true';
} else {
echo 'false';
}
?>
In this example, the script receives the username value, performs the validation, and echoes ‘true’ or ‘false’ depending on the validation result.
What happens if the AJAX request fails or the server-side script encounters an error?
If the AJAX request fails or the server-side script encounters an error, the jQuery Validation Plugin treats it as a validation failure, and the field will be marked as invalid. You can handle AJAX errors by using the ‘error’ option in the AJAX settings. For example:$("#myForm").validate({
rules: {
username: {
remote: {
url: "check-username.php",
type: "post",
data: {
username: function() {
return $("#username").val();
}
},
error: function() {
alert("An error occurred while validating the username.");
}
}
}
}
});
In this example, if an error occurs during the AJAX request, an alert message will be displayed.
Can I use the remote rule to validate multiple fields at once?
No, the remote rule is designed to validate one field at a time. If you need to validate multiple fields at once, you should create a separate server-side script for each field and use the remote rule for each field separately.
Can I use the remote rule with dynamic data or fields that change?
Yes, you can use the remote rule with dynamic data or fields that change. The remote rule sends an AJAX request every time the field value changes and loses focus, so it can handle dynamic data. However, keep in mind that this could result in a large number of AJAX requests if the field value changes frequently.
Can I use the remote rule without the jQuery Validation Plugin?
No, the remote rule is a feature of the jQuery Validation Plugin and cannot be used without it. If you need to perform AJAX validation without the plugin, you can use jQuery’s AJAX methods directly, but you will need to handle the validation logic and error messages yourself.
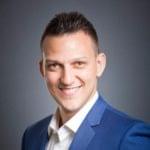
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.