Keep up to date on current trends and technologies
Ruby - Heroku
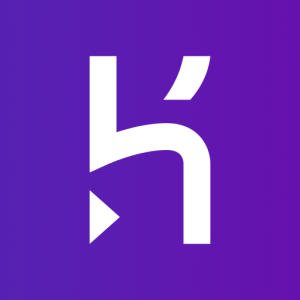
Leverage Heroku’s Metrics for Better App Performance
Vinoth
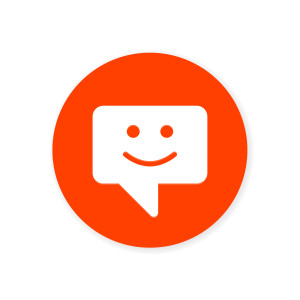
Create a Chat App with Rails 5, ActionCable, and Devise
Ilya Bodrov-Krukowski
Build a Sinatra API Using TDD, Heroku, and Continuous Integration with Travis
Darren Jones
Nitrous.IO and Heroku: A Perfect Pair
Thom Parkin
Deploying a Yeoman/Angular app to Heroku
Brad Barrow
Get Started with Sinatra on Heroku
Jagadish Thaker
Showing 6 of 6