- What is a Git merge, and how does it work?
- What are the benefits of using Git merge for integrating code?
- What are the potential risks or challenges of using Git merge?
- How can I resolve conflicts that may arise during a Git merge?
- Are there any best practices or guidelines for using Git merge in a team environment?
- Conclusion
- Frequently Asked Questions (FAQs) about Git Merge
As a web developer, it is imperative to know how to use Git, a popular version control system, to manage code and collaborate with other developers. One of the most important features of Git is Git merge, which allows you to combine changes from different branches of your codebase. In this tutorial, we’re going to dive deep into Git merge, its benefits, potential risks, and best practices for using it in a team environment.
What is a Git merge, and how does it work?
When you’re working in a team, it is common for multiple developers to be working on the same codebase at the same time. These developers may work on different features, bug fixes, or enhancements, and eventually, their changes will need to be combined into a single, stable version of the codebase. That’s where Git merge comes in.
Git merge is a command that allows you to combine changes from one branch with another branch. When you merge two branches, Git will create a new commit that includes the changes from both branches. Git tries to merge changes automatically, but sometimes you may encounter conflicts that need to be resolved manually.
Let’s take a look at an example. Suppose you’re working on a feature branch called feature-branch
, and your colleague is working on a bug fix branch called bug-fix-branch
. Both branches have some changes, and you want to merge them to create a new branch called new-branch
.
To merge feature-branch
and bug-fix-branch
, you can use the following command:
$ git merge feature-branch bug-fix-branch
Git will try to merge the changes from feature-branch
and bug-fix-branch
automatically. If there are no conflicts, Git will create a new commit that includes the changes from both branches.
What are the benefits of using Git merge for integrating code?
Git merge comes with several benefits, including:
1. Better collaboration: Git merge allows multiple developers to work on the same codebase simultaneously, without having to worry about conflicts.
2. Easier code management: Git merge allows developers to manage changes to a codebase easily. Instead of having many different versions of the same code, merging allows all changes to be compiled into a single, stable version.
3. Easy rollback: Suppose that after the merge, the code is not working as expected. In that case, Git allows developers to easily revert back to the previous version using the git revert
command.
What are the potential risks or challenges of using Git merge?
While Git merge is beneficial, there are some potential risks as well. These include:
1. Conflicts: Sometimes, Git may not be able to merge changes automatically, and conflicts may arise. These conflicts need to be resolved manually, which can be time-consuming and sometimes complicated.
2. Regression errors: During the merge, it is essential to ensure that the codebase is stable and does not introduce any new errors or bugs. It is essential to test thoroughly after a merge.
3. Mistaken merge: Accidentally merging the wrong branches can lead to severe issues that can be tough to fix. It is essential to verify carefully before merging any two branches.
How can I resolve conflicts that may arise during a Git merge?
Suppose Git merges fail to automatically merge branches. In that case, you may need to resolve conflicts manually. The following steps will help you to resolve conflicts that arise during git merge:
Step 1: First, run the git status
command to see which files have conflicts:
$ git status
On branch new-branch
You have unmerged paths.
(fix conflicts, and run "git commit")
This output shows that there are conflicts in the branch. The next step is to open the file with conflicts.
Step 2: Open the file with conflicts using a text editor. In the file, search for the conflicts. The conflicts start with <<<<<<< HEAD
and end with >>>>>>>
.
<<<<<<< HEAD
This is the code that you wrote.
=======
This is the code that your colleague wrote.
>>>>>>> branch-name
Step 3: Edit the file to remove the conflicts. You can select which code to keep or merge both codes together.
This is the code that you wrote.
This is the code that your colleague wrote.
Step 4: Save the file and run the following command:
$ git add file-name
Step 5: Commit the changes using the following command:
$ git commit -m "Resolved conflicts in file-name"
Git will create a new commit that includes the changes from both branches, and the conflicts will be resolved.
Are there any best practices or guidelines for using Git merge in a team environment?
Git merge is essential when working in a team environment, and some best practices and guidelines can help you avoid risks and potential problems. These include:
1. Create short-lived branches: Instead of working directly on the main branch, create short-lived feature or bug fix branches that can be merged back into the main branch once the work is complete.
2. Regularly merge code changes: Integrating changes regularly can prevent conflicts and reduce the chance of regression errors.
3. Test thoroughly: Always test your code thoroughly after merging, and ensure that the new code is working correctly and does not introduce any new bugs.
4. Use descriptive commit messages: Use clear, concise commit messages that accurately describe the changes made in each commit.
5. Verify before merging: Double-check to ensure that you are merging the correct branch, and the codebase is stable before merging.
Conclusion
Git merge is a critical feature of Git, and it is essential to understand how it works, as well as its benefits and potential risks. By following the guidelines and best practices, you can use Git merge effectively to manage code changes and collaborate with other developers in a team environment.
Frequently Asked Questions (FAQs) about Git Merge
What is the difference between ‘git merge’ and ‘git rebase’?
Both ‘git merge’ and ‘git rebase’ are used to integrate changes from one branch into another. However, they do it in different ways. ‘Git merge’ takes the contents of a source branch and integrates it with the target branch. On the other hand, ‘git rebase’ moves or combines a sequence of commits to a new base commit. Essentially, it’s like saying “I want to base my changes on what everybody else has done.” In a team setting, ‘git rebase’ can be more useful than ‘git merge’ as it can make your feature branch up to date with the latest code from the main branch.
How can I resolve merge conflicts in Git?
Merge conflicts occur when competing changes are made to the same line of a file, or when one person edits a file and another person deletes the same file. The git merge command will fail and Git will ask you to fix the problem. You can do this by opening the file and looking for the conflict markers (<<<<<<, ======, and >>>>>>). The changes from the HEAD or base branch are in the top part (between <<<<<< and ======), and the changes from the merging branch are in the bottom part (between ====== and >>>>>>). You need to manually choose which version to keep.
What does ‘git merge –abort’ do?
The ‘git merge –abort’ command is used to stop the merge process and return to the state before the merge began. This is useful when you realize that the merge you started is more complex than you initially thought, or if there are too many conflicts and you decide to abort the merge process.
How can I merge a specific commit in Git?
If you want to merge a specific commit into your current branch, you can use the ‘git cherry-pick’ command followed by the commit hash. This command applies the changes introduced by the named commit on the current branch. It’s like a series of git apply commands: it applies changes introduced by the commit you specify, as a patch, onto your current branch.
What is a fast-forward merge in Git?
A fast-forward merge can occur when there is a linear path from the current branch tip to the target branch. Instead of creating a new commit, Git just moves the branch pointer forward to the latest commit. This type of merge keeps the commit history linear and clean.
How can I undo a ‘git merge’?
If you want to undo a ‘git merge’, you can use the ‘git reset’ command with the ‘–hard’ option and provide the hash of the commit you want to go back to. This will destroy all changes and bring your branch back to the exact state it was in when the specified commit was made.
What is the difference between ‘git merge’ and ‘git pull’?
Git pull’ is a combination of ‘git fetch’ and ‘git merge’. When you use ‘git pull’, Git will get all the changes from the remote repository, then try to merge it into your current branch. ‘Git merge’ is a command that is used to merge branches or integrate changes from another branch without having to fetch the changes first.
How can I merge two branches without a commit?
If you want to merge two branches without creating a merge commit, you can use the ‘git merge’ command with the ‘–no-commit’ option. This will perform the merge but will not automatically create a new commit. You can then make any changes or adjustments before manually committing the merge.
What is a ‘git merge’ strategy?
A ‘git merge’ strategy is a method that Git uses to combine different sequences of commits into one unified history. Git offers several merge strategies with ‘recursive’ being the default one. Other strategies include ‘resolve’, ‘octopus’, ‘ours’, and ‘subtree’. Each strategy is useful in different scenarios and can be specified with the ‘-s’ or ‘–strategy’ option when performing a merge.
How can I avoid a merge commit in Git?
If you want to avoid a merge commit while merging two branches, you can use the ‘git rebase’ command before performing the merge. This will move all changes to the tip of the branch that is being merged, resulting in a fast-forward merge and avoiding a merge commit.
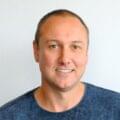
Mark Harbottle is the co-founder of SitePoint, 99designs, and Flippa.