The Backtick Character
You’ve no doubt run into this situation: you have a line of text, maybe HTML, that extends over several lines. How to put those multiple lines in HTML? It is possible to use the + operator to join strings across multiples lines (provided you enclose them in quotes) or even just append the line with the backslash character ("\"
). Those work. They are also awkward, error prone, and ugly.
ES6 introduces another way to extend strings across a single line, using the back-tick character (`
). At its simplest, this solves two issues with one throw – the aforementioned one of letting you have one string with embedded carriage returns, and the not to be sneezed at ability to incorporate both single and double quotes in a string without having to escape them.
Consider this code and observe what it generates below:
`"This isn't just another quote," Ada said. "It's a magical quote.";
"I'm not quite sure what you mean."
Clive settled onto the couch, one foot over the armchair rest, eyeing her dubiously.
"Consider this - if I have to write a quote in code normally, say something like 'This is a weird quote.' \
then I have to spend a lot of time using escape characters like \\" and \\', and they can make for real \
legibility problems. With a magic quote, though, they just go away!"
"Really?"
"You do have to double escape your escape characters, however. \\n will still get interpreted as an hard \
carriage return, but \\\\n will give you the slash followed by the letter 'n'."
`
console.log(quote)
This generates the output:
“This isn’t just another quote,” Ada said. “It’s a magical quote.”
“I’m not quite sure what you mean.”
Clive settled onto the couch, one foot over the armchair rest, eyeing her dubiously.
“Consider this – if I have to write a quote in code normally, say something like ‘This is a weird quote.’ then I have to spend a lot of time using escape characters like \"
and \'
, and they can make for real legibility problems. With a magic quote, though, they just go away!”
“Really?”
“You do have to double escape your escape characters, however. \n
will still get interpreted as a hard carriage return, but \\n
will give you the slash followed by the letter 'n'
.”
The effect is instantaneous here – in effect, the backtick (or more accurately, the template literal operator) performs a conversion of carriage returns, tabs and quotes into their respective normal string representations. This not only handles the pain of dealing with apostrophes in conversational text (as demonstrated above), but it also makes the corresponding code considerably easier to read.
Note as well that the terminating backspace character is still recognized within templates, which can be very useful when you have text that runs longer than your available screen-width. The difference between a regular string and a template string is that in the former you need to escape every line, while in the latter you need only escape the overly long strings.
If your string contains a number of escaped characters (such as the newline or tab character) and you don’t want to get caught up in counting slashes, you can also use the String.raw` literal form.
String.raw`The sequence \t puts a tab into a template literal`
“The sequence \t puts a tab into a template literal.”
Evaluating Expressions in Template Strings
Template literals also solve another problem: they can evaluate variables within the string. The${expr}
construct, when used within a template literal, will evaluate the expression and incorporate this into a string itself.
var name = "Ada";
var vocation = "steampunk programmer";
varpcAssert = `${name} is a ${vocation}.`;
console.log(pcAssert)
// "Ada is a steampunk programmer."
Using a template literal as a…template
In the example below, the variable name and vocation are both predefined, and the${}
expressions then replace the variables with their associated values. In a similar vein, you can both evaluate content and use object references within these expressions:
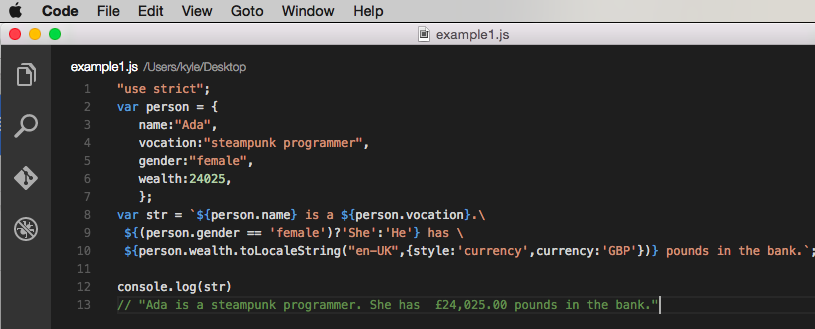
Template Functions
At this stage, template literals are beginning to look interesting. You can create a complex template literal that can reduce the amount of time embedded such content into a string. However, template literals begin to come into their own when combined with functions which produce the same output.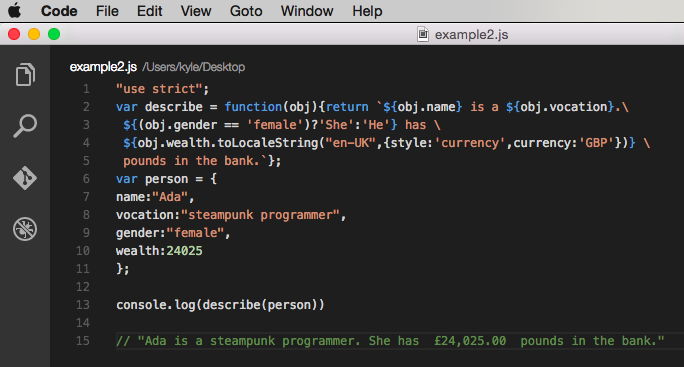
var pounds = (amount) => (
amount.toLocaleString("en-UK", {
style:'currency',
currency:'GBP'
})
);
varpronounUCase = (gender) => (gender == 'female') ? 'She' : 'He';
var describe = (obj) => (
`${obj.name} is a ${obj.vocation}.\
${pronounUCase(obj.gender)} has ${pounds(obj.wealth)} \ in the bank.`
);
var person = {
name: "Ada",
vocation: "steampunk programmer",
gender: "female",
wealth: 24025
};
console.log(describe(person))
// "Ada is a steampunk programmer. She has £24,025.00 in the bank."
If the goal was to make a method on person
, you’d be better off going with the more traditional functional notation, as the this keyword is not defined within an arrow function:
var person = {
name: "Ada",
vocation: "steampunk programmer",
gender: "female",
wealth: 24025,
pounds: (amount) => (amount.toLocaleString("en-UK", {
style: 'currency',
currency:'GBP'
})
),
pronoun: (gender) => (gender === "female") ? "She" : "He",
toString: function(){
varself = this;
return (`${self.name} is a ${self.vocation}.\
${self.pronoun(self.gender)} has ${self.pounds(self.wealth)} \
in the bank.`
)
}
};
console.log(""+ person)
// "Ada is a steampunk programmer.She has £24,025.00 in the bank."
Creating a toString() Method with a Template
The use of the toString() method should be noted, astoString()
is automatically called whenever an object is converted into a string without having to be explicitly invoked. This means that while person
itself will always give the object, "" + person
will return the templated string as output, making toString()
useful for defining on classes and prototyped objects.
Building HTML and XML Templates
Such template functions have obvious implications for building HTML and XML structures. For instance, you can construct a list of links in HTML by passing an array into a template function to generate an HTML string, then inserting this into another element:var li = (obj) => `<li><a href="${obj.url}" target="_new">${obj.label}</a></li>`;
var ul = (arr) => `<ul>${arr.map((obj) => li(obj)).join('\n')}</ul>`;
var arr = [
{url: "http://www.twitter.com", label: "Twitter"},
{url: "http://www.linkedin.com", label: "Linked In"},
{url: "http://www.facebook.com", label: "Facebook"}
];
document.getElementById('displayList').innerHTML = ul(arr);
Creating lists of links using templates.
This will generate the following output:<ul>
<li><a href="http://www.twitter.com" target="_new">Twitter</a></li>
<li><a href="http://www.linkedin.com" target="_new">LinkedLinked Linked In</a></li>
<li><a href="http://www.facebook.com" target="_new">Facebook</a></li>
</ul>
which will then be rendered as an unordered list of links.
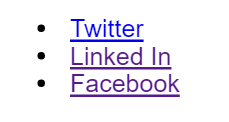
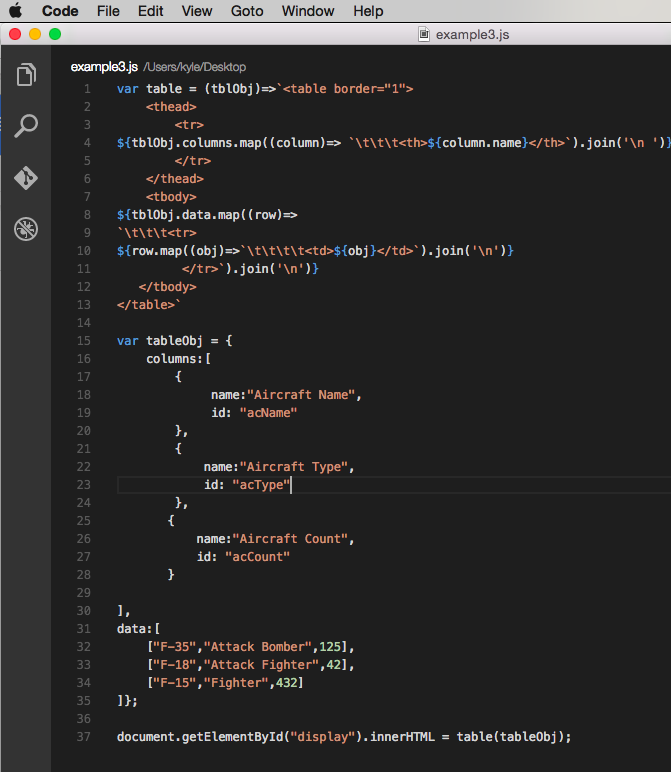
Aircraft Name | Aircraft Type | Aircraft Count |
---|---|---|
F–35 | Attack Bomber | 125 |
F–18 | Attack Fighter | 42 |
F–15 | Fighter | 432 |
Frequently Asked Questions (FAQs) about ES6 Template Literals
What are the benefits of using ES6 template literals over traditional string concatenation?
ES6 template literals offer several advantages over traditional string concatenation. Firstly, they provide a more readable and concise syntax. You don’t need to use the ‘+’ operator to concatenate strings, which can make your code cleaner and easier to understand. Secondly, template literals allow you to embed expressions within your strings. This means you can insert variables, perform calculations, or call functions directly within your strings. Lastly, template literals support multi-line strings without needing to use escape characters or concatenation, making it easier to work with large strings or HTML templates.
Can I use template literals with older versions of JavaScript?
Template literals are a feature of ES6, also known as ECMAScript 2015. Older versions of JavaScript do not support template literals. However, you can use transpilers like Babel to convert your ES6 code into ES5 syntax that can be understood by older browsers. It’s important to note that while this allows you to use ES6 features, it may not perfectly replicate all functionality.
How do I embed expressions within a template literal?
To embed expressions within a template literal, you use the ${} syntax. You can place any valid JavaScript expression within the curly braces, and it will be evaluated and inserted into the string. For example, let name = 'John'; console.log(
Hello, ${name}!);
would output “Hello, John!”.
Can I use template literals for multi-line strings?
Yes, one of the major benefits of template literals is their support for multi-line strings. In traditional JavaScript, you would need to use the ‘\n’ escape character or concatenate multiple strings together. With template literals, you can simply create a new line by pressing ‘Enter’, and the new line will be preserved in the resulting string.
Are there any performance differences between template literals and string concatenation?
In most cases, the performance difference between template literals and string concatenation is negligible and unlikely to impact your application. However, in some browsers, template literals may be slightly slower than string concatenation due to the additional processing required to parse and evaluate the template literal. Despite this, the improved readability and functionality of template literals often outweighs any minor performance considerations.
Can I nest template literals within each other?
Yes, you can nest template literals within each other. This can be useful when you want to create complex strings with multiple levels of interpolation. However, keep in mind that nested template literals can become difficult to read and maintain, so they should be used sparingly.
How do tagged template literals work?
Tagged template literals are an advanced feature of template literals that allow you to parse and manipulate the string and substitutions before they are combined. A tag is simply a function that is called with the string parts and substitutions as its arguments. This can be used for a variety of purposes, such as sanitizing user input, localization, or custom string formatting.
Can I use template literals with HTML templates?
Yes, template literals are a great fit for working with HTML templates. Their support for multi-line strings and embedded expressions makes it easy to create dynamic HTML. You can insert variables directly into your HTML, and even include logic or loop through arrays to generate complex HTML structures.
Are there any security concerns with using template literals?
Like any feature that allows you to embed expressions within strings, template literals can potentially be a security risk if misused. If you are inserting user input into a template literal, you should always sanitize the input to prevent cross-site scripting (XSS) attacks. However, this is a general best practice and not a specific weakness of template literals.
Can I use template literals in Node.js?
Yes, template literals are fully supported in Node.js as of version 4.0.0. This means you can use them in your server-side JavaScript code just as you would in the browser.

Kurt Cagle consults for DevelopIntelligence. He lives in Issaquah, Washington and works as a technology evangelist and writer.