Building robust websites and applications isn’t just writing semantic HTML and fancy CSS. It’s also important to keep an eye on web performance from the beginning of your projects. There are many techniques that can help with this, and thus create a better user experience. Creating CSS sprites is one of them. Using this technique you combine all of your small images (sprites) into a larger image (sprite sheet) along with CSS to position it.
You can generate your sprites using different tools, yet in this article I’ll show you how to achieve it using Compass. Before that, let me introduce you to the project that will be used for this article.
Setting up a project
To better demonstrate how Compass’s sprite builder works, I set up a new project. Here’s the structure of it:
Compass-Sprites/
├── config.rb
├── index.html
├── stylesheets/
│ ├── ie.css
│ ├── print.css
│ └── screen.css
├── sass/
│ ├── ie.scss
│ ├── print.scss
│ └── screen.scss
└── images/
└── icons/
├── arrow-down.png
├── facebook-active.png
├── facebook-hover.png
├── facebook.png
├── twitter-active.png
├── twitter-hover.png
├── twitter.png
└── zuckerberg.png
Notice, there’s an icons
folder within the images folder, which contains all the images that will be used for this project.
You can grab all the files for this project by downloading this zip file.
Basic Spriting
Creating a sprite sheet is a 4-step process:
- Import the required Sass assets (variables, mixins, functions) from the corresponding module (Utilities).
- Import all the images (sprites) that are in the
icons
folder. - Include the Sass mixin that will generate the CSS classes for the sprites.
- Add the generated classes to the related HTML elements.
To be more specific, in the screen.scss
file we add the following directives:
@import "compass/utilities/sprites";
@import "icons/*.png";
@include all-icons-sprites;
Again, notice that icons
is the name of the folder containing the images.
As long as we save and compile the screen.scss
file the sprite sheet will be created in the images
folder. The name of the sprite sheet would look like this:
icons-s82ea51d311.png
The name of it retains the following convention: <Images_Folder_Name>-<Cache_Buster>
. The cache buster changes every time we update the sprite sheet. In this way, the browser will always have and use the latest version of it.
The initial sprite sheet of our project will look something like this:
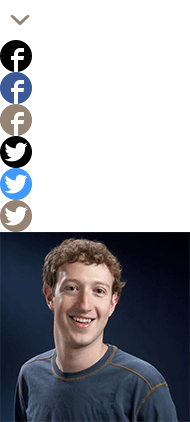
And here are the CSS classes that Compass generates for us:
.icons-sprite,
.icons-arrow-down,
.icons-facebook,
.icons-twitter,
.icons-zuckerberg {
background-image: url('../images/icons-s82ea51d311.png');
background-repeat: no-repeat;
}
The names of the classes above have the following convention: <Images_Folder_Name>-<Image_Name>
.
For each image, Compass applies a different background position. For example, here’s the background-position
for the zuckerberg.png
image:
.icons-zuckerberg {
background-position: 0 -232px;
}
At this point we add the generated classes to the corresponding HTML elements.
Here’s the element that is related to the zuckerberg.png
image:
<h1 class="icons-zuckerberg">Mark Zuckerberg</h1>
Keep in mind that Compass API can generate a sprite sheet only for PNG images.
Magic Selectors
Compass has the ability to create classes for different states using the sprite magic selectors.
For instance, in our project there’s the facebook.png
image. Let’s say that we want to have different background colors for the images for the hover
and active
states. In this case, we add the facebook-hover.png
and facebook-active.png
images. The names of these images should follow the convention: <Image_Name>-<State>
.
After that, Compass generates the following CSS classes:
.icons-facebook:hover, .icons-facebook.facebook-hover {
background-position: 0 -72px;
}
.icons-facebook:active, .icons-facebook.facebook-active {
background-position: 0 -40px;
}
Customizing the Generated Classes
In the previous sections, we saw how Compass can generate the CSS classes. But, what if we want to customize the names of these classes? Hopefully, we can do it using the <Images_Folder_Name>-sprite(Image_Name)
mixin, instead of the all-<Images_Folder_Name>-sprites
mixin.
To illustrate this, let’s assume that we want to change the name of the icons-zurckerberg
class. In the screen.scss
file we add the following class:
.creator {
@include icons-sprite(zuckerberg);
}
This class is derived as follows:
<Desired_Class_Name> {
@include <Images_Folder_Name>-sprite(Image_Name);
}
And here’s the CSS that is created and can be applied to the element we want:
.creator {
background-position: 0 -232px;
}
Notice that the only thing that changes is the name of the class attribute. The CSS properties have the same values as the first mixin.
The advantage of the second mixin is that it offers you the option to create your custom classes. However, this might not be a good solution if your project has a lot of images.
Configuration Options
Compass allows us to customize the sprite sheet using additional configuration variables. Here are some of them:
$sprite-selectors
: Determines the states for which the magic sprite selectors are enabled. Possible values:hover
,target
,active
,focus
. Default values:hover
,target
,active
,focus
.$disable-magic-sprite-selectors
: Determines if the sprites will contain magic selectors. Possible values:true
orfalse
. Default value:false
.$icon-layout
: Determines the layout of sprites in the sprite sheet. Possible values:horizontal
,vertical
,diagonal
,smart
. Default value:horizontal
.$icon-spacing
: Determines, in pixels, the amount of spacing between each sprite. Default value:0
.$sprite-dimensions
: Determines if the generated CSS classes will contain the dimensions of each sprite. Default value:false
.$default-sprite-separator
: Determines the default separator for the sprites. Possible values:-
or_
. Default value:-
.
The variables above should be defined before creating our sprite sheet. That means, before adding the following directive:
@import "icons/*.png";
Let’s now experiment with two of them in our project.
As a first attempt, we change the sprite sheet layout to smart
adding the following variable:
$icons-layout:smart;
This results in the following sprite sheet:
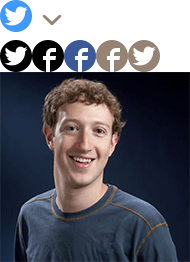
It’s worth mentioning that we can’t use the smart
method and the $icon-spacing
variable at the same time. This happens because the method arranges the sprites in an optimal way removing as much white space between them as possible.
Now let’s see what happens when we add the variable below:
$icons-sprite-dimensions:true;
When compiled we get something like this CSS output for one of the generated classes:
.icons-facebook {
height: 32px;
width: 32px;
}
So what’s happened? Compass has the ability to measure the dimensions of each image and output them in the compiled CSS. This is a great feature, which could be useful when the sprite sheet contains images that have different dimensions. However, if we have images with same dimensions this could result in bloated CSS.
Beyond the Basics
As we have previously seen, creating a sprite sheet requires the following magic directives:
@import "<Images_Folder_Name>/*.png";
@include all-<Images_Folder_Name>-sprites;
@include <Images_Folder_Name>-sprite(Image_Name);
Beyond them, there are also some extra functions and mixins we can use to create our sprite sheet. Here are some of them:
sprite-map
: A function that creates a CSS sprite map containing the images.sprite
: A mixin that returns the image and thebackground-position
of it.sprite-dimensions
: A mixin that returns the dimensions of the sprite.
To better understand how these work, let’s again assume that we want to create a custom class with name creator
. We define the sprite map (requesting a smart
layout) and get the background-position
of the icons-zurckerberg.png
image. Then, we retrieve the dimensions of this image. After, we’re ready to assign this class to the desired HTML element.
Here’s the code that invokes what is described above:
$icons: sprite-map("icons/*.png", $layout: smart);
.creator {
background: sprite($icons, zuckerberg);
@include sprite-dimensions($icons, zuckerberg);
}
And here’s the CSS:
.creator {
background: url('../images/icons-s855a77086a.png') 0 -232px;
height: 190px;
width: 190px;
}
Conclusion
In this article, I briefly introduced you to the concept behind the CSS sprites technique. Then, I showed you the basics of Compass’s sprites generator. There are more options than we have covered here, but I hope you got the idea of generating sprites with Compass.
Frequently Asked Questions (FAQs) about CSS Sprites with SASS and Compass
What are the benefits of using CSS sprites with SASS and Compass?
CSS sprites are a technique in web design where a group of images are combined into one image to reduce the number of server requests. This can significantly improve the performance of a website. SASS and Compass are tools that make it easier to work with CSS sprites. They automate the process of generating sprite images and CSS, saving you time and reducing the chance of errors. Additionally, they provide a more organized and maintainable codebase, making it easier to update and modify your sprites in the future.
How do I create a CSS sprite using SASS and Compass?
To create a CSS sprite with SASS and Compass, you first need to install Compass and create a new project. Then, place all the images you want to include in your sprite in a folder within your project. In your SASS file, import the Compass sprite module and use the sprite-map function to generate your sprite image and CSS. You can then use the sprite-url, sprite-position, and sprite-dimensions functions to control the display of individual images from your sprite.
Can I control the spacing between images in a CSS sprite with SASS and Compass?
Yes, you can control the spacing between images in a CSS sprite with SASS and Compass. This is done using the $spacing argument in the sprite-map function. By default, Compass places a 10px gap between images to prevent bleeding, but you can adjust this to any value you like.
How do I update a CSS sprite with SASS and Compass?
Updating a CSS sprite with SASS and Compass is straightforward. Simply add, remove, or modify the images in your sprite folder, then recompile your SASS file. Compass will automatically generate a new sprite image and update the CSS accordingly.
Can I use CSS sprites with SASS and Compass in a responsive design?
Yes, you can use CSS sprites with SASS and Compass in a responsive design. However, it’s important to note that sprite images are not inherently responsive. You’ll need to use additional CSS techniques, such as media queries and flexible image techniques, to ensure your sprites display correctly on different screen sizes.
How do I handle retina displays when using CSS sprites with SASS and Compass?
Handling retina displays when using CSS sprites with SASS and Compass involves creating two versions of your sprite image – one for standard displays and one for retina displays. You then use media queries in your CSS to serve the appropriate image based on the resolution of the user’s device.
Can I use CSS sprites with SASS and Compass in a CSS grid layout?
Yes, you can use CSS sprites with SASS and Compass in a CSS grid layout. The sprite-position function can be used to control the position of individual images from your sprite within grid items.
How do I debug issues with my CSS sprite in SASS and Compass?
Debugging issues with your CSS sprite in SASS and Compass can be done using browser developer tools. You can inspect the generated CSS and sprite image to ensure they are correct. If you’re having trouble, it can be helpful to temporarily add borders or background colors to your sprite images to see exactly how they are being positioned.
Can I use CSS sprites with SASS and Compass in a CSS flexbox layout?
Yes, you can use CSS sprites with SASS and Compass in a CSS flexbox layout. The sprite-position function can be used to control the position of individual images from your sprite within flex items.
How do I optimize my CSS sprite for performance with SASS and Compass?
Optimizing your CSS sprite for performance with SASS and Compass involves a few steps. First, ensure you are only including images in your sprite that are actually used in your design. Second, try to use the smallest possible dimensions for your images without sacrificing quality. Finally, consider compressing your sprite image using a tool like TinyPNG to reduce its file size.

George is a freelance web developer and an enthusiast writer for some of the largest web development magazines in the world (SitePoint, Tuts+). He loves anything related to the Web and he is addicted to learning new technologies every day.