In this article, we will explore a unique way of allowing your customers to easily redeem a product or group of products they may have paid for already perhaps at a conference or other similar event.
Let’s talk a bit more about the concept. Say you are a store owner with a revolutionary new product and you present it to thousands of people at a conference. At the end of your speech, those customers willing to try your product can do so and pay for it ahead of time. You may even have enticed them by lowering your price to the first 500 customers.
In this scenario, what you’ll do in WooCommerce is create 500 coupons, with a product discount of 100%. You could use the Smart Coupons plugin to generate these 500 coupons so that you don’t have to create them manually. Each customer who paid in advance gets a coupon code but as far as the customer knows it is just a code to redeem the products.
Creating the Coupon Codes
If you are serious enough about your offer, then you will try to make the coupon codes look random and make it hard, if not impossible for users to come up with a valid coupon code. Make sure you select which products are tied to this coupon so we can add them to the cart automatically later on. Take a look at one of the coupons I created, pay close attention to the settings:
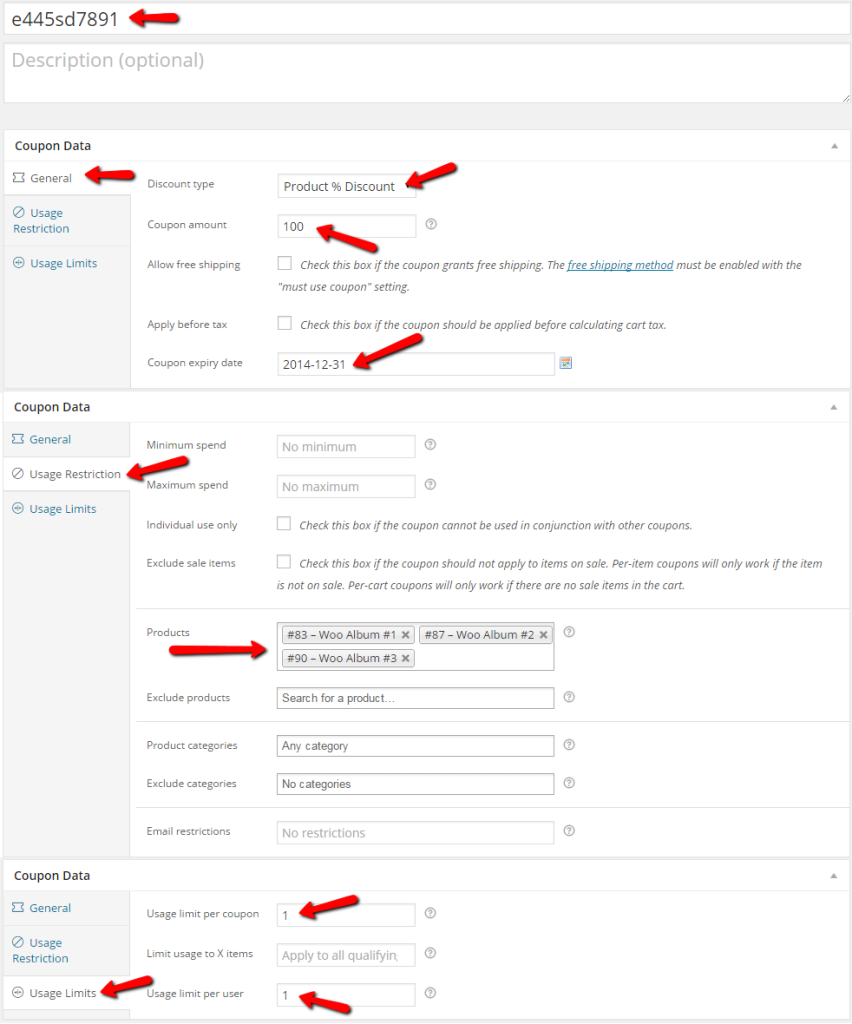
Creating the WooCommerce Redeem Products Page
You can easily make a copy of your page.php and turn it into a page template so you can use it for the page we are going to be sending those customers so they can redeem their products. Name it something like page-coupon-redeem.php
The following markup is what we’ll use to format the form displayed to the customer on that page. It is just a form with two fields, one for entering their code and the actual submit button. We are trying to keep this as simple as possible for the customer; so we are going to do everything via Ajax so there are as little page loads as possible.
<div class="redeem-coupon">
<form id="ajax-coupon-redeem">
<p>
<input type="text" name="coupon" id="coupon"/>
<input type="submit" name="redeem-coupon" value="Redeem Offer" />
</p>
<p class="result"></p>
</form><!-- #ajax-coupon-redeem -->
</div><!-- .redeem-coupon -->
When the user enters a code and hits the submit button, the value entered in the text field is sent for validation and if it happens to be valid, then the user will be redirected to the ‘Cart’ page and the products will already be there to checkout for the price of $0. If by any chance the code is incorrect, then we notify the user that something is wrong and the code entered is not valid.
Building the Ajax Functionality
If you have never done Ajax in WordPress, please refer to my previous article Adding Ajax to Your WordPress Plugin for a brief introduction to how Ajax is performed in WordPress.
Let’s begin building the Ajax functionality required for our ‘Redeem Your Products Page’ to function as expected. All the code that follows goes in your functions.php file of your theme.
Register Our Ajax Handler
First register our Ajax call handler by hooking into the wp_ajax_$action
and wp_ajax_nopriv_$action
actions.
add_action( 'wp_ajax_spyr_coupon_redeem_handler', 'spyr_coupon_redeem_handler' );
add_action( 'wp_ajax_nopriv_spyr_coupon_redeem_handler', 'spyr_coupon_redeem_handler' );
Note that the same function is handling the Ajax call for both customers whether they are logged in or not.
Next, we are going to start building our logic to account for the following possible scenarios:
- Code text field being empty
- Code being invalid, meaning is not a valid coupon code
- Successfully presenting a valid coupon
Handling the Coupon Logic
Now that we have our actions registered and we know what to do, we need to write the actual function which will handle our possible scenarios.
<?php
function spyr_coupon_redeem_handler() {
// Get the value of the coupon code
$code = $_REQUEST['coupon_code'];
// Check coupon code to make sure is not empty
if( empty( $code ) || !isset( $code ) ) {
// Build our response
$response = array(
'result' => 'error',
'message' => 'Code text field can not be empty.'
);
header( 'Content-Type: application/json' );
echo json_encode( $response );
// Always exit when doing ajax
exit();
}
// Create an instance of WC_Coupon with our code
$coupon = new WC_Coupon( $code );
// Check coupon to make determine if its valid or not
if( ! $coupon->id && ! isset( $coupon->id ) ) {
// Build our response
$response = array(
'result' => 'error',
'message' => 'Invalid code entered. Please try again.'
);
header( 'Content-Type: application/json' );
echo json_encode( $response );
// Always exit when doing ajax
exit();
} else {
// Coupon must be valid so we must
// populate the cart with the attached products
foreach( $coupon->product_ids as $prod_id ) {
WC()->cart->add_to_cart( $prod_id );
}
// Build our response
$response = array(
'result' => 'success',
'href' => WC()->cart->get_cart_url()
);
header( 'Content-Type: application/json' );
echo json_encode( $response );
// Always exit when doing ajax
exit();
}
}
Handling the Form Submission with jQuery
All that’s left to do now is build the jQuery code to submit the coupon code to WordPress for processing and handling the JSON data returned.
jQuery( document ).ready( function() {
jQuery( '#ajax-coupon-redeem input[type="submit"]').click( function( ev ) {
// Get the coupon code
var code = jQuery( 'input#coupon').val();
// We are going to send this for processing
data = {
action: 'spyr_coupon_redeem_handler',
coupon_code: code
}
// Send it over to WordPress.
jQuery.post( woocommerce_params.ajax_url, data, function( returned_data ) {
if( returned_data.result == 'error' ) {
jQuery( 'p.result' ).html( returned_data.message );
} else {
// Hijack the browser and redirect user to cart page
window.location.href = returned_data.href;
}
})
// Prevent the form from submitting
ev.preventDefault();
});
});
Final Result
The styling of the form is entirely up to you. I’ve used the default Twenty Twelve theme and WooCommerce’s dummy data and with a few CSS rules this is what I’ve got below.
Empty Field Error Message
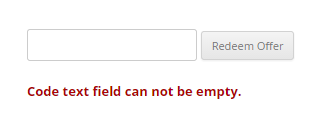
Invalid Code Error Message
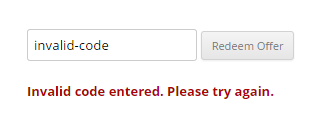
Valid Code/Cart Populated
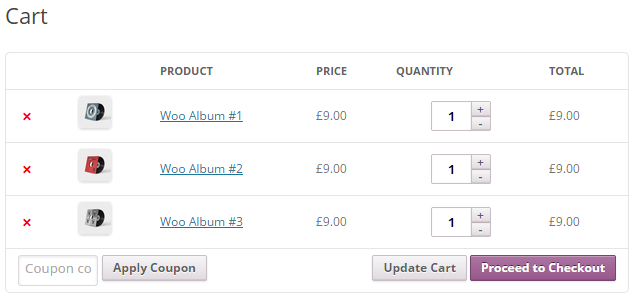
Conclusion
Even though this scenario might not apply to every store out there, WooCommerce shines in providing us with a set of tools through their API so that we can accomplish almost anything we set our minds to. Add WordPress to the mix and you’ve got a complete eCommerce solution which is second to none.
It is my hope that through this article, I’ve provided some insight of how coupons work in WooCommerce and that you’ll feel more comfortable in using it on your next project.
Frequently Asked Questions (FAQs) on Creating a WooCommerce Redeem Coupon Page
How Can I Create a Unique Coupon Code in WooCommerce?
Creating a unique coupon code in WooCommerce is a straightforward process. First, navigate to the WooCommerce section of your WordPress dashboard. Click on ‘Coupons’ under the ‘Marketing’ tab. Click on ‘Add Coupon’ and you’ll be directed to a new page where you can create your unique coupon code. You can customize the coupon code, discount type, coupon amount, and other settings according to your needs. Remember to click ‘Publish’ to save your new coupon code.
Can I Set an Expiry Date for My WooCommerce Coupon?
Yes, you can set an expiry date for your WooCommerce coupon. When creating or editing a coupon, you’ll find an ‘Coupon expiry date’ option under the ‘General’ tab. Here, you can select the date you want the coupon to expire. After setting the date, remember to click ‘Publish’ or ‘Update’ to save your changes.
How Can I Limit the Usage of My WooCommerce Coupon?
WooCommerce allows you to limit the usage of your coupons. Under the ‘Usage limit’ tab when creating or editing a coupon, you can set a limit on the number of times the coupon can be used, the number of items it can apply to, or the number of times a single user can use the coupon. After setting the limits, remember to click ‘Publish’ or ‘Update’ to save your changes.
Can I Apply a Coupon to Specific Products or Categories?
Yes, WooCommerce allows you to apply coupons to specific products or categories. Under the ‘Usage restriction’ tab when creating or editing a coupon, you can select specific products or categories that the coupon will apply to. You can also exclude certain products or categories from the coupon’s discount. After setting the restrictions, remember to click ‘Publish’ or ‘Update’ to save your changes.
How Can I Enable Customers to Apply Coupons Automatically?
To enable customers to apply coupons automatically, you’ll need to use a plugin like ‘Smart Coupons for WooCommerce’. Once installed and activated, navigate to the plugin’s settings. Here, you can enable the ‘Auto Apply’ option, which will automatically apply the coupon’s discount when the customer’s cart meets the coupon’s conditions.
Can I Create a Coupon That Gives a Free Gift?
Yes, you can create a coupon that gives a free gift. You’ll need to use a plugin like ‘Smart Coupons for WooCommerce’. Once installed and activated, navigate to the plugin’s settings. Here, you can create a new coupon and set the discount type to ‘Free Gift’. You can then select the product that will be given as a free gift when the coupon is used.
How Can I Share My WooCommerce Coupon with Customers?
There are several ways to share your WooCommerce coupon with customers. You can include the coupon code in your marketing emails, display it on your website, or share it on social media. You can also use a plugin like ‘Smart Coupons for WooCommerce’ to send the coupon directly to customers via email.
Can I Track the Usage of My WooCommerce Coupon?
Yes, you can track the usage of your WooCommerce coupon. Under the ‘Reports’ section of your WooCommerce dashboard, you can view detailed reports on the usage of your coupons. You can see how many times each coupon has been used, the total discount amount given, and more.
Can I Create a Coupon That Applies to Shipping Costs?
Yes, you can create a coupon that applies to shipping costs. When creating or editing a coupon, under the ‘Discount type’ dropdown, select ‘Shipping discount’. You can then set the discount amount. Remember to click ‘Publish’ or ‘Update’ to save your changes.
Can I Restrict a Coupon to New Customers Only?
Yes, you can restrict a coupon to new customers only. Under the ‘Usage restriction’ tab when creating or editing a coupon, you can check the box that says ‘Allow new customers only’. This will ensure that only customers who are making their first purchase can use the coupon. After setting the restriction, remember to click ‘Publish’ or ‘Update’ to save your changes.
Yojance Rabelo has been using WordPress to build all kinds of Websites since July 2006. In his free time he likes to try out new technologies, mainly PHP and JavaScript based, and also playing with his EV3RSTORM, a Lego MINDSTORMS robot. He maintains his own blog among other sites about various topics.