var interval = setInterval(function() {
console.log('i');
}, 1000);
console.log(interval);
It you can see it assigns a random number as the id for the event. Then when we clear the interval it uses that id.
Simply by calling:
clearInterval(interval);
A Diirty Solution
Now this is not good coding practice (for a number of reasons) but if you really need to clear that interval we can simply do a loop to find the id of the event to stop all setInterval() events that are running. I’ve done a quick jsfiddle to show you. https://jsfiddle.net/WqCws/.
for(i=0; i<100; i++)
{
window.clearInterval(i);
}
I’m pretty sure this will also work for setTimeout() and clearTimeout();
Frequently Asked Questions (FAQs) about clearInterval in JavaScript
What is the purpose of clearInterval in JavaScript?
The clearInterval function in JavaScript is used to stop or clear a timer that was previously established by setInterval. The setInterval function allows you to execute a specific function or code snippet at specified intervals (in milliseconds). However, it will continue to run indefinitely until the page is refreshed or closed, or until clearInterval is called. By passing the ID value returned by setInterval to clearInterval, you can stop the timer and prevent the function or code snippet from being executed again.
How do I use clearInterval without knowing the ID?
Normally, clearInterval requires the ID returned by setInterval to clear the timer. However, if you don’t have the ID, you can still clear all intervals by creating a loop that calls clearInterval for all possible IDs within a certain range. This method is not recommended for regular use because it can inadvertently clear intervals that you want to keep running. It’s best to always store and manage your interval IDs so you can clear them individually when needed.
Can I clear all intervals at once in JavaScript?
Yes, you can clear all intervals at once in JavaScript. However, this is not a built-in feature of the language. You would need to manually create a loop that calls clearInterval for all possible IDs within a certain range. This method is not recommended for regular use because it can inadvertently clear intervals that you want to keep running. It’s best to always store and manage your interval IDs so you can clear them individually when needed.
What happens if I call clearInterval with an invalid ID?
If you call clearInterval with an ID that doesn’t correspond to an active interval, nothing will happen. The function will simply do nothing and return undefined. This is because clearInterval only works on IDs that have been returned by setInterval and have not yet been cleared.
Can I use clearInterval in a function?
Yes, you can use clearInterval inside a function. In fact, this is a common practice in JavaScript programming. You might set up an interval inside a function, store the ID in a variable, and then use that variable to clear the interval later on, either inside the same function or in a different function.
How does clearInterval work with multiple intervals?
If you have multiple intervals running, you can clear them individually by calling clearInterval with their respective IDs. Each call to setInterval returns a unique ID, so you can store these IDs in separate variables and use them to clear the intervals as needed.
Can I use clearInterval in a loop?
Yes, you can use clearInterval in a loop. This is often done when you want to clear multiple intervals at once. You would loop through all possible IDs within a certain range and call clearInterval for each one. However, this method is not recommended for regular use because it can inadvertently clear intervals that you want to keep running.
What is the difference between clearInterval and clearTimeout?
Both clearInterval and clearTimeout are used to stop timers in JavaScript, but they work with different types of timers. clearInterval is used to stop intervals, which are timers that execute a function or code snippet at regular intervals. clearTimeout, on the other hand, is used to stop timeouts, which are timers that execute a function or code snippet once after a specified delay.
Can I use clearInterval with other JavaScript functions?
Yes, you can use clearInterval with any JavaScript function that is being executed at regular intervals using setInterval. You simply need to pass the ID returned by setInterval to clearInterval to stop the function from being executed again.
Is there a performance impact when using clearInterval?
Using clearInterval itself does not have a significant impact on performance. However, if you have a large number of intervals running at the same time, it can slow down your JavaScript execution and potentially cause performance issues. In such cases, it’s important to manage your intervals properly and clear them when they are no longer needed.
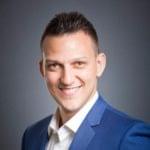
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.