- A bit of an over-react-ion
- Just tell me what React is, please
- All I want for JSXmas is you
- Virtual DOM, DOM, DOM, DOM, DOM
- I don’t know art, but I know what I like
- You ‘n’ I flow in the same direction
- Flummoxed by Redux
- I will never change for you
- Tell me which way to go
- Get that baton round
- Last night shallow rendering changed my life
- Build it and they will come
- I want to B-abel to use ES2015
- Isomorphic server side rendered universal JavaScript
- Gah, I’ve got lint in my code…ooh and some chewing gum
- A used case
- End of the beginning
If you’re thinking of using React but are overwhelmed by the number of resources, have given up resisting the noise, or just want to keep abreast of the state of things, read on. If you already use React, hate React, still want to resist React…read on anyway for cliff notes on this weekend’s gathering.
A bit of an over-react-ion
There is probably nothing that can be written on the subject that hasn’t already been covered. Reading every resource in the awesome react repo is equivalent to completing the whole Encyclopaedia Britannica cannon. Time-consuming, impressive and enlightening. However, we only have until Saturday to avoid embarrassment and a coded tutorial isn’t going to cover all the buzzwords likely to crop up.
Just tell me what React is, please
Ben Backbone says, “It’s just the V in MVC so it’s only 1/3 as good”.
You say, “I find comparisons to MVC unhelpful nor intuitive, I play with Lego and focus on making beautiful blocks that can fit anywhere”.
This is a building block:
({ someText }) => <div>{ someText }</div>
React is not a framework, it’s a library which allows you to compose these building blocks to build maintainable interactive interfaces.
Now you’re probably wondering why React is so daunting and why the scroll bar is far higher than you were hoping…well, like camping, you can’t just go into the woods with your bivvy, you need this…and this…and this…
All I want for JSXmas is you
Alan Angular says, “You’re putting HTML in your JS and are mixing concerns”.
You say, “You’re drunk Alan, go home…but also, it’s a delightful way to express markup that can be transpiled to many things, looks like HTML so there’s no new DSL I have to learn, and really my only concern is presenting a view of my data”.
Yes JSX has probably taken the brunt of hatred, but it’s what really set React apart and has now been adopted by other libraries. No more low level fiddling with the DOM, checking for attributes or worrying about cross browser quirks. I can write something that eerily looks like HTML but I can also use good old JS to manipulate it.
Virtual DOM, DOM, DOM, DOM, DOM
Edward Ember says, “The Glimmer engine repaints are far faster than the Virtual DOM”.
You say, “While performance is a concern for me I very rarely/never build apps that need to render 10k ever changing list items. If the tech ticks the community, stability, maintainability, testability boxes, it’s probably not dog slow.”.
Interacting with the DOM is comparably slow, in that it usually takes up the most amount of time in a given operation compared to JS in memory. The React team developed the Virtual DOM (VDOM) to allow them to make a fast comparison of state changes in order to minimize the amount of slow work that needs to be done.
I don’t know art, but I know what I like
Charlotte CSS says, “Inline styles are everything that’s wrong with the internet. No :before/:after, patchy @media print support, un-DRY code, mixing concerns [Alan +1], extra overhead for :hover etc. need I go on?”.
You think, “This all pales in comparison to the fact they don’t even work with a strict content security policy *, glad she didn’t mention that”.
You say, “That’s quite a lot of hyperbole before desert. Pseudo selectors are a hack anyway, I prefer not to use them, I like trees, DRY is what I don’t like about your blanket CSS and once again, I’m just concerned with using my presentation layer for it’s intended purpose”.
Ok, so you don’t have to use inline styles with React, you can be less bold and try CSS Modules (if you enjoy long class names in your HTML), one of the many CSS inlining tools or just use BEM.
I strongly suggest you give it a go though, it means your component’s content and style is tightly coupled (which is what you want) and can be dropped in anywhere with confidence. Developers don’t accidentally tread on each other’s feet and there’s no ungainly naming convention that must be taught and has to be policed. JS is also great at doing the heavy lifting for animations.
* to avoid this ever so slight wrinkle you can use the Shadow DOM, take a look at react-shadow or maple for ideas.
You ‘n’ I flow in the same direction
Kevin Knockout says, “With two way data binding you get highly interactive interfaces with little boilerplate code”.
You say, “A unidirectional data flow is half the headache, debugging and testing in one direction is much more pleasurable, especially with pure functions that have no side effects”.
So we have our building blocks written in what looks like HTML, with their own protected styles, and they will only update the DOM when they have to…but how do you make them show stuff? By using idempotent render functions with referential transparency that are as pure as un-driven snow of course ;-)
If you only remember one thing for the party, it’s this core concept, React components are just functions. If you give them the same input, they should return the same output, you pass this data via props. However, components can also maintain their own state, which should be treated with kid gloves.
In a React application you ideally want one source of truth, smart components (which know about data and how to pass it on) and dumb components (which know nothing and do as they’re told). The majority of your application should be made of dumb components, the worker bees, with higher order components orchestrating data to pass to them.
Flummoxed by Redux
Jeremy jQuery says, “The problem with React is, it takes you three days to try out fifteen Flux implementations to come to the conclusion that you don’t really know which one is best. You go with one and it takes another day to write what I did in 10 mins”.
You say, “Yeah, it was a bit of a gem from Facebook (FB), leaving it to the community to thrash out a vague philosophy, I completely agree. Although, now I have my Flux implementation, my code is easy to reason about, testable and can scale”.
The beauty of the open source community (OSC) is that an optimal solution will eventually rise up, so suggesting philosophies to the OSC is no bad thing. FB themselves do Flux in many different ways, so what is it?
In essence it’s the mechanics of the unidirectional flow of data we covered. A view performs an action, which by way of a dispatcher updates a store that updates your higher order components and subsequent children. Always one way, always predictable.
Earlier this year, a cheeky chappy called Dan put something together for a talk. People liked it, it got more stars on GitHub than the Milky Way and so he worked on it full time. It’s called Redux and it’s the implementation I recommend. It’s not completely Flux but the dev tooling is exceptional with time travel and undo/redo etc. out of the box thanks to store updates only through pure reducers.
I will never change for you
People are too busy with the port and cheese at this juncture but if they were to ask about immutability here’s a high level view.
When state changes in our React application everything is checked for prop differences before another check is made for DOM differences. Making deep equality checks is expensive and so is diffing the VDOM. Fortunately we can lend React a helping hand by using immutable data. If a component’s props have not changed and they are an immutable object, the current prop value and the next prop value will have the same reference that means we can perform a quick shallow equality check in shouldComponentUpdate. This member of the React life cycle will cut out the VDOM diffing saving work and therefore time.
With Redux’s reducers you get this concept out of the box, you take in a previous state and you create a brand new state based on an action’s payload. This is the second benefit of immutability and a recurring theme; it makes your application predictable. No bugs because something accidentally changed something else it wasn’t meant to, or had business with.
Tell me which way to go
Edward Ember says, “I can scaffold out my project in a couple of minutes and have absolutely everything I need, I find React too lightweight”.
You say, “For me using lots of libraries/building blocks that each do one thing well (UI microservices) is highly maintainable and easy to future proof”.
So React doesn’t come with a router, but Michael Jackson does. This is the de facto way of routing in React and a virtual requirement for any single page application (SPA). We now have Promises, the fetch API, service workers, web workers, local storage, JWT, intl etc. etc. You can build an SPA natively with very little need for large frameworks* beyond a few polyfills.
* not that frameworks are bad per se
Get that baton round
You say, “I’ve got all I need for this SPA, my mug is empty, this article is too long with no discernible tl;dr, I’m going to sort out my dry cleaning before Saturday”.
I say, “I tell you what to say, not the other way round but…there’s one last optional piece and then we need to talk about how you’re going to put all this together”.
Redux goes to great lengths to explain how to perform asynchronous actions and seed your app with data through various means including middleware. Neal Netflix pops up and says, “Let’s chill and have a look at Falcor“, you say, “Actually that sounds rather good but I’m going to give Relay and GraphQL a little go first”.
FB had a problem when they were building mobile native apps, mobile web apps and their other suite of web products. How can you co-ordinate incredibly complex data syncing across these platforms and use the same language when REST isn’t cutting it? Thus we have GraphQL (nothing to do with Graph databases), which much like Flux is just a concept with quite a few implementations (including JS).
GraphQL is a language that allows the front-end developer to control their data requirements. No customized REST end points, no bothering the various back end teams for an extra field to be sent, just ask for a fragment of data with optional parameters and it will be returned to you.
Relay then hooks up your React components by way of containers and routes to your GraphQL end point. This rather complex marriage results in optimistic updates, query batching and data sync happiness out of the box…as long as you have a GraphQL server…and schema…and the ability to get your head around Relay mutations and the funky query language…
Last night shallow rendering changed my life
Roberto Reacto says, “Facebook use Jest to test their components and so that’s what I use”.
You say, “It’s super slow, it’s overly complicated. It really loves to mock everything. Shallow render and test all the things”.
If FB really does use Jest, I can only commend their patience but you’ll rarely see it mentioned on the conference circuit. When I read this, I had an epiphany and the rest is history.
This is the best way to test the front-end. No DOM, very few dependencies, just crack out Mocha and Assert and you’ll have rock solid, super fast unit tests that you can rely on. You’ll get a long way by testing the various moving parts, which just leaves some lightweight functional smoke testing to confirm interaction, nice.
Build it and they will come
Graham Grunt gulps after grazing on a cold piece of broccoli and says, “How do you build your app without build tools?”.
You say, “With node, npm scripts and a module loader, my workflow is greatly reduced yet incredibly powerful”.
Another pivotal read for me was this, I then use webpack as my weapon of choice but jspm and Browserify are perfect alternatives, or anything that gives you:
- Hot reloading: because we’re super lazy devs and time is money
- Babel integration: for reasons that we’ll get onto
- Minification, asset/vendor management, resource hashing: because you want one tiny lump of cache busted JS to run your beautiful app
I want to B-abel to use ES2015
Oscar Old-School says, “I’ve been using ES5 since 2009, legacy browsers don’t support ES2015, classes in JS!? The spec changes yearly, staged propositions like Object.observe get dropped while you’re using them, Babel drops support for well-used features and what’s with the three dots…?”.
You say, “For someone so old school you’re remarkably familiar with all these things and you make some good points. As you mentioned Babel, you know that we can transpile down to ES5 which runs in all modern browsers. You don’t have to use every feature in ES2015 and if you use proposed features you’re asking for trouble. The three dots are also awesome, spread the love”.
So, formerly of 6to5 fame, when it was written by a kid in his history class (until he found out Dolly was working three fewer hours), Babel is the go to JS transpiler, unless you prefer more Gallic tools.
It’s not a requirement (like TypeScript isn’t for Angular 2 ;-)), but writing all your code in ES2015 (stage proposals for side projects) will just make your day that much better. Once you start destructuring you’ll never want to stop.
And yes, classes in principle are best avoided, composition over inheritance is preferred, but…if you only extend once and it makes your React components more readable using idiomatic native code…perhaps we just go with it…
Isomorphic server side rendered universal JavaScript
Ally Anderson says, “JavaScript is everything that’s wrong with the internet. These dynamic sites are not SEO optimized and how many nested elements do you want!?”.
You say, “True, JS shouldn’t be used for everything, but the things I build require interaction and great UX, I still care about graceful degradation and progressive enhancement. This is why I render as much content as I can server side with semantic elements and form posts which gives me SEO for free”.
Usually when people use isomorphic and universal they mean server side rendering, which React is aimed at, splitting out its main engine from react-dom and react-dom/server.
As a small caveat, do not blindly render server side because the time to glass is faster. Depending on the situation this might not be the case when it comes to round trips, latency, the browser and hardware etc. etc.
Gah, I’ve got lint in my code…ooh and some chewing gum
No need for debate around this one, there’s general consensus around the table and much nodding (even from Alan), linting is imperative to collaboration on a shared code base. Time shouldn’t be wasted during code reviews on semi-colons and indentations; they should be about intent and architecture.
Now, there is a difference between code quality and code style, quality focusing on code best practice and style focusing on…styling the code, with semi-colons and the like. You can split these jobs out with JSCS and JSHint for example but the current darling of the linting world is ESLint.
For React I use Airbnb’s config which uses this for their Reacty bits. Stick this in your posttest script and merge PRs like they’re going out of fashion.
A used case
Sally Static says, “I wouldn’t use React for a static site, there’s no real interaction and I get everything I need from Jekyll“.
You say, “Have you tried Gatsby? Are you sure you don’t want live reloading in your life?”.
It’s possible to argue that using React for everything is overkill, if you don’t need interaction and the VDOM via 42kb of JS, you shouldn’t use it. However…React is not solely about the VDOM, it’s about testable styled Lego bricks. If you’re doing anything that’s slightly more than trivial and you don’t mind writing a bit of JS, I would advocate using React*.
* or any other library with a component philosophy but this article is about the merits of React ;-)
End of the beginning
React comes with a massive community and ecosystem to complement the single responsibility library, which means you can just drop a component into your existing site, something not possible with a behemoth framework, or write a whole app from the ground up.
React has taken over the world1, WordPress is going all React2, you must use React3. If you’re looking for a dependable unopinionated component library that does one thing well, then hopefully this article has provided you with enough links and words to google/sitepoint for the next month.
It’s like coding in the 90s with whole page refreshes (albeit optimized client side ones), inline styles (albeit not a requirement) and inline events (albeit one root event)…and “HTML in your JS”, but there’s nothing wrong with that4.
- it hasn’t
- it’s not…yet
- you don’t, but it’s a good idea to ;-)
- there’s a lot wrong with the 90s, this conclusion uses a liberal creative licence
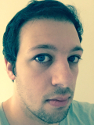
I'm a full-stack JavaScript developer and full-time pedant. I enjoy making great web products with the latest technologies. I've been known to attend the odd pub quiz in my spare time.