Here is a quick note of AJAX before jQuery 1.8 and the newer version. Don’t forget .success() and .error() still supported in jQuery 1.9.1 so it won’t break your old code and plugins you use. I have also drawn up some some New jQuery.ajax() Examples jQuery 1.9+ so check them out!
Deprecation Notice: The jqXHR.success(), jqXHR.error(), and jqXHR.complete() callbacks are deprecated as of jQuery 1.8. To prepare your code for their eventual removal, use jqXHR.done(), jqXHR.fail(), and jqXHR.always() instead.
Get HTML using AJAX before jQuery 1.8
$.ajax({
url: 'test.html',
dataType: 'html',
success: function (data, textStatus, xhr)
{
console.log(data);
},
error: function (xhr, textStatus, errorThrown)
{
console.log('error: '+textStatus);
}
});
Get HTML using AJAX jQuery 1.8+
// cache: false is used to fetch the latest version
$.ajax({
url: "test.html",
cache: false
})
.done(function(data, textStatus, jqXHR)
{
console.log(data);
})
.fail(function(jqXHR, textStatus, errorThrown)
{
console.log('error: '+textStatus);
});
Mutiple callbacks can be specified for an $.ajax() request. Callback methods .done(), fail(), always(), then.() are all promise methods of the jqXHR object. All these callback methods fire once the $.ajax() process terminates. Promise callbacks are invoked in the order they are registered.
Frequently Asked Questions (FAQs) about AJAX and jQuery 1.8
What is the significance of beforeSend in AJAX and jQuery 1.8?
The beforeSend option in AJAX and jQuery 1.8 is a pre-request callback function that can be used to modify the jqXHR (in jQuery 1.4.x, XMLHTTPRequest) object before it is sent. It allows you to manipulate the request before it is sent to the server. This can be useful for adding headers or setting other options on the request. If the beforeSend function returns false, the request will not be sent.
How can I use AJAX async with beforeSend?
The async option in AJAX is used to specify whether the request should be handled asynchronously or not. If set to true, the JavaScript will continue to execute while the request is being processed. The beforeSend option can be used in conjunction with async to modify the request before it is sent, even when the request is asynchronous.
How can I fire a certain action before and after every AJAX call?
You can use the beforeSend and complete options in AJAX to fire actions before and after every AJAX call. The beforeSend function will be called before the request is sent, and the complete function will be called after the request is completed, regardless of whether it was successful or not.
What is the difference between beforeSend and .before in jQuery?
The beforeSend option in AJAX is a callback function that is called before the request is sent. The .before method in jQuery is used to insert content before each element in the set of matched elements. They serve different purposes and are used in different contexts.
How can I handle errors in AJAX and jQuery 1.8?
You can use the error option in AJAX to handle errors. This is a function that is called if the request fails. It is passed three arguments: the jqXHR object, a string describing the type of error that occurred, and an optional exception object if one occurred.
How can I send data with an AJAX request in jQuery 1.8?
You can use the data option in AJAX to send data with the request. This can be a plain object or a string. If it is a plain object, it is converted to a string before it is sent. If it is a string, it is sent as is.
How can I specify the type of data that I expect back from the server?
You can use the dataType option in AJAX to specify the type of data that you expect back from the server. This can be “xml”, “json”, “script”, “html”, or “text”. The default is “intelligent guess”, which means jQuery will try to infer the type of data based on the MIME type of the response.
How can I make a POST request with AJAX in jQuery 1.8?
You can use the type option in AJAX to specify the type of request to make. If you set this to “POST”, a POST request will be made. You can then use the data option to send data with the request.
How can I abort an AJAX request in jQuery 1.8?
You can abort an AJAX request by calling the .abort() method on the jqXHR object that is returned by $.ajax(). This will immediately terminate the request.
How can I use AJAX to load data into a specific element?
You can use the .load() method in jQuery to load data from the server and place it into a specific element. This method uses AJAX behind the scenes to make the request. You simply pass the URL of the resource you want to load and a callback function to execute when the request is complete.
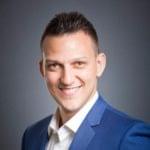
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.