Media queries are the core technology behind Responsive Web Design yet, despite a plethora of options, few of us dare venture beyond min-width
(and possibly max-width
), i.e.
/* mobile-first layout */
.column {
width: 100%;
}
/* tablet layout */
@media (min-width: 50em) {
.column {
float: left;
width: 50%;
}
}
/* desktop layout */
@media (min-width: 75em) {
.column {
width: 33.33%;
}
}
Few other media query properties are required for general use:
width
,height
and thedevice
declarations are a little too exactingresolution
can be used for high-density Retina screens but the new HTML5picture
element andsrcset
attribute are more appropriate for image contentcolor
andmonochrome
properties may only be useful when targeting e-readersaural
,braille
,handheld
,projection
,tv
,scan
,grid
etc. have specific device use casesaspect-ratio
andorientation
may only be needed when screens fall outside the standard 4:3 and 16:9 range
min-height
and max-height
properties? If we only consider min-width
and/or max-width
we may be making life more difficult for those using smaller devices. There are several situations when vertical media queries can be useful…
1. Managing Fixed Elements
Does your hamburger icon have its own fixed navigation bar at the top of the screen? Perhaps it’s only 50 pixels, but that could be 10% of the screen on a smaller device or those held in landscape orientation. The user will require more scrolling and find it more difficult to use. Therefore, consider fixing the bar only when the height becomes practical, e.g./* static nav bar */
nav {
width: 100%;
height: 50px;
color: #fff;
background-color: #333;
}
/* fixed nav bar */
@media (min-height: 800px) {
body {
padding-top: 75px;
}
nav {
position: fixed;
top: 0;
left: 0;
}
}
Admittedly, this means users have to scroll back to the top of the page to access navigation. For longer pages, consider a less obtrusive fixed element on smaller screens, e.g.
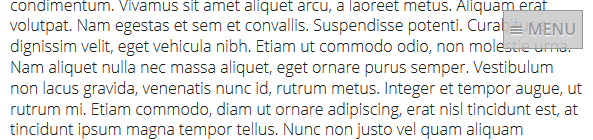
2. Managing “The Fold”
Yes, the fold is dead. The fold is irrelevant to developers and designers; there is no standard screen size, resolution, browser chrome or window height. Unfortunately, it’s not necessarily irrelevant to individual users. Consider a web page header containing a carousel with a height of 600px. Anyone visiting that page with similar browser dimensions may not realize there is content below — it looks as if the page has neatly ended. They could miss important calls-to-action or further information. It’s difficult to guarantee the fold won’t affect everyone but there are options. In this example, screens under 500px will show a full-height 600px carousel. The cropped element should make it obvious there’s more content. Between 500px and 800px, the carousel shrinks to 400px so the user can see more content below. At 800px, the carousel returns to 600px but, again, extra content can be seen..carousel {
width: 100%;
height: 600px;
}
@media (min-height: 500px) {
.carousel { height: 400px; }
}
@media (min-height: 800px) {
.carousel { height: 600px; }
}
This technique is unlikely to work in all situations but, at the very least, you could use vertical media queries to ensure call-to-action buttons always appear on-screen without having to scroll.
3. Adjusting Font Sizes and Weights
Smaller fonts can be used on smaller screens. This need not reduce legibility because the device is normally held closer than a monitor. Even if you choose not to change the body text, those large 24px headings can almost certainly be downsized. This will reduce the scrolling required and is especially useful when the user holds their device in landscape orientation.4. Adjusting Line Heights and Margins
Similarly, text spacing can be tighter on smaller screens. For example:h1 {
font-size: 1.25rem;
font-weight: normal;
line-height: 1.2;
margin: 1em 0 0 0;
}
p {
font-size: 0.75rem;
line-height: 1.2;
margin: 0 0 1em 0;
}
@media (min-height: 50em) {
h1 {
font-size: 2rem;
font-weight: bold;
margin: 1.5em 0 0.5em 0;
}
p {
font-size: 1rem;
line-height: 1.5;
}
}
5. Adjusting Content Visibility
On smaller height screens, you could consider making content ‘optional’. Perhaps you could implement an accordion for some sections or question/answer blocks. At larger heights, all the content could become visible without having to click a heading first. That said, be wary about completely hiding content. If a widget or feature isn’t useful to someone using a smaller device, why is it necessary to show it on a larger screen? Whilemin-height
and max-height
will never be used to the same extent as min-width
and max-width
they provide a number of design opportunities which we’re only just beginning to exploit.
Frequently Asked Questions (FAQs) on Vertical Media Queries
What are the benefits of using vertical media queries in web design?
Vertical media queries are a powerful tool in responsive web design. They allow designers to create layouts that adapt to the height of the viewport or device screen. This is particularly useful for devices with different screen sizes and orientations. For instance, a vertical media query can be used to adjust the layout when a user switches from portrait to landscape mode on a mobile device. It can also be used to ensure that content is always visible and accessible, regardless of the viewport height.
How do I implement vertical media queries in CSS?
Implementing vertical media queries in CSS is straightforward. You use the @media rule followed by the condition for the media feature. For example, to apply styles for devices with a viewport height of 800px or less, you would write:@media (max-height: 800px) {
/* styles go here */
}
This block of CSS will only be applied when the condition inside the parentheses is true.
Can I use vertical media queries to target specific devices?
Yes, you can use vertical media queries to target specific devices based on their screen height. However, it’s generally recommended to design for the content and not for specific devices. This is because device specifications can vary greatly and new devices are constantly being released.
What is the difference between min-height and max-height in vertical media queries?
The min-height and max-height media features are used to apply styles based on the height of the viewport. Min-height is used when you want styles to apply to viewports that are at least a certain height, while max-height is used when you want styles to apply to viewports that are at most a certain height.
How can I combine horizontal and vertical media queries?
You can combine horizontal and vertical media queries by using the ‘and’ keyword. For example, to apply styles for devices with a maximum width of 600px and a maximum height of 800px, you would write:@media (max-width: 600px) and (max-height: 800px) {
/* styles go here */
}
This block of CSS will only be applied when both conditions are true.
What are some common use cases for vertical media queries?
Vertical media queries are commonly used to adjust layout for different screen orientations, to ensure that content is always visible and accessible, and to create more immersive user experiences on devices with large screens.
Can vertical media queries be used with other CSS features?
Yes, vertical media queries can be used in conjunction with other CSS features such as Flexbox and Grid to create complex, responsive layouts.
Are there any limitations or drawbacks to using vertical media queries?
One potential drawback to using vertical media queries is that they can add complexity to your CSS. It’s also important to note that media queries do not override other CSS rules, but add to them. This means that you need to ensure your media queries are properly structured to avoid unexpected results.
How do vertical media queries affect performance?
Vertical media queries themselves have minimal impact on performance. However, the CSS inside the media query can affect performance if it’s complex or if there are a large number of media queries.
How can I test my vertical media queries?
You can test your vertical media queries using the developer tools in your web browser. Most browsers allow you to simulate different screen sizes and orientations, making it easy to see how your layout responds to different conditions.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.